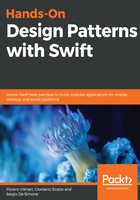
上QQ阅读APP看书,第一时间看更新
Using weak
Using weak will ensure that we never create a strong reference in ref, but will not retain the objects, either. If the object passed in ref is never retained by any other object, it will automatically be deallocated. This will lead to unexpected behavior, as the chain will be deallocated, and only a returned object will be kept in the memory:
class MemoryLeak {
weak var ref: MemoryLeak?
init(ref: MemoryLeak) {
self.ref = ref
}
init() {
ref = self
}
}
func test() -> MemoryLeak {
let a = MemoryLeak()
let b = MemoryLeak(ref: a)
let c = MemoryLeak(ref: b)
a.ref = c
return a
}
let result = test()
assert(result.ref != nil)
In the preceding code, we changed the MemoryLeak class, in order to keep a weak reference in ref. Unfortunately, the program will crash at the assertion line, as the ref property will be deallocated.
This is often the behavior that you are looking for with delegation. Using weak for the delegate lets you safely avoid thinking about the potential reference cycle; however, the delegates should be retained on their own.