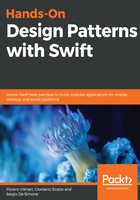
Dangling pointers
Dangling pointers are the opposite of over-retained objects. You can encounter dangling pointers when trying to access a deallocated object. This is best illustrated by the following example:
- (void) doSomething {
MyObject *myObject = [[MyObject alloc] init]; // retainCount is +1
[myObject release]; // retain count is 0; object is deallocated
// More things happening in this method
[myObject myMethod];
}
This may not cause a crash all of the time, as myObject could be properly set to nil. When it crashes, you'll face the dreaded NSInvalidArgumentException, which, upon first glance, doesn't make much sense:
*** Terminating app due to uncaught exception 'NSInvalidArgumentException', reason: '-[<insert a type here> myMethod]: unrecognized selector sent to instance 0x12345679
We never have a call to myMethod on any other type. This crash often means that the contents of the memory at the myObject address have been replaced by some other object. Because we called release too early, the memory has been reclaimed, and potentially replaced by another object.
In order to overcome the burden of writing the manual reference counting, Apple introduced ARC. In the next section, we'll look at what this compiler feature can do, in order to provide efficient memory management.