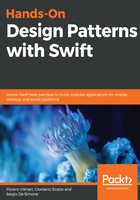
上QQ阅读APP看书,第一时间看更新
Generic types
You can also make complex types generic. In our example, we created this wrapper around a list of Runnable, called ManyRunner. The job of a many runner is to run all of the runnables. The ManyRunner is itself Runnable, so we have created a kind of type recursion, as follows:
struct ManyRunner<T>: Runnable where T: Runnable {
let runnables: [T]
func run() {
runnables.forEach { $0.run() }
}
}
Let's also provide a base object that runs a simple Incrementer. Each time the Incrementer is run, the static count will increment, to keep track of the number of invocations:
struct Incrementer: Runnable {
private(set) static var count = 0
func run() {
Incrementer.count += 1
}
}
When using generics on types, remember that the types have to be the same:
// This works
let runner = ManyRunner(runnables: [Incrementer(),Incrementer()])
runner.run()
assert(Incrementer.count == 2)
// runner is of type ManyRunner<Incrementer>
ManyRunner(runnables: [Incrementer(), Runners(runnables: [Incrementer()])] as [Runnable]).run()
// This produces the following compile error
// In argument type '[Runnable]', 'Runnable' does not conform to expected type 'Runnable'
We'll look at how to overcome these limitations in Chapter 8, Swift-Oriented Patterns.