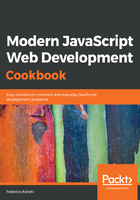
Spreading and joining values
A new operator, ..., lets you expand an array, string, or object, into independent values. This is harder to explain than to show, so let's see some basic examples:
// Source file: src/spread_and_rest.js
let values = [22, 9, 60, 12, 4, 56];
const maxOfValues = Math.max(...values); // 60
const minOfValues = Math.min(...values); // 4
You can also use it to copy arrays or concatenate them:
// Source file: src/spread_and_rest.js
let arr1 = [1, 1, 2, 3];
let arr2 = [13, 21, 34];
let copyOfArr1 = [...arr1]; // a copy of arr1 is created
let fibArray = [0, ...arr1, 5, 8, ...arr2]; // first 10 Fibonacci numbers
You can also use it to clone or modify objects; in fact, this is a usage we're going to meet again later, in Chapter 8, Expanding Your Application, mostly when we use Redux:
// Source file: src/spread_and_rest.js
let person = { name: "Juan", age: 24 };
let copyOfPerson = { ...person }; // same data as in the person object
let expandedPerson = { ...person, sister: "María" };
// {name: "Juan", age: 24, sister: "María"}
This is also useful for writing functions with an undefined number of arguments, avoiding the old style usage of the arguments pseudo-array. Here, instead of splitting an element into many, it joins several distinct elements into a single array. Note, however, that this usage only applies to the last arguments of a function; something such as function many(a, ...several, b, c) wouldn't be allowed:
// Source file: src/spread_and_rest.js
function average(...nums: Array<number>): number {
let sum = 0;
for (let i = 0; i < nums.length; i++) {
sum += nums[i];
}
return sum / nums.length;
};
console.log(average(22, 9, 60, 12, 4, 56)); // 27.166667