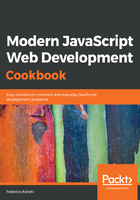
Getting started
Flow will not check every file unless you expressly require it to. For a file to be checked, you must add a simple comment to the very top, as shown in the following code snippet. Flow will ignore any files that lack this comment, so even if you were adding the tool to an already existing project, you could do it gradually, adding files one at a time:
/* @flow */
Starting with Flow's controls, you just have to specify what data type you expect any variable to be, and Flow will check that it's always used correctly. Fortunately, Flow is also capable of determining data types by value; for example, if you assign a string to a variable, it will assume that this variable is meant to contain strings. Adapting an example from https://flow.org/en/docs/usage/, you could write the following:
/* @flow */
function foo(x: ?number): string {
if (x) {
return x;
} else {
return "some string";
}
}
console.log(foo("x"));
The :?number and :string annotations specify that x is an optional numeric parameter, and that foo should return a string. Can you see two problems with the rest of the code? If you use npm run flow, you'll get a report showing what the problem is. First, you cannot return x, because of the data types mismatch between the variable and the expected return value:
Error ------------------------------------------------------------------------------------- src/types_examples.js:5:16
Cannot return x because number [1] is incompatible with string [2].
2│
[1][2] 3│ function foo(x /* :?number */) /* :string */ {
4│ if (x) {
5│ return x;
6│ } else {
7│ return 'some string';
8│ }
Second, you are trying to call a function but passing a parameter of the wrong type:
Error------------------------------------------------------------------------------------- src/types_examples.js:12:17
Cannot call foo with 'x' bound to x because string [1] is incompatible with number [2].
[2] 3│ function foo(x /* :?number */) /* :string */ {
:
9│ }
10│
11│ // eslint-disable-next-line no-console
[1] 12│ console.log(foo('x'));
13│
All of the preceding code is (except for the type declarations) valid JS, so it would have been accepted; Flow tells you about the problems so that you can fix them. Now, let's get into greater detail, and see all of the possibilities that this tool gives us.