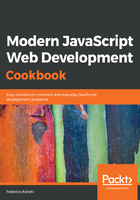
Adding code quality checks with ESLint
JS is a very potent language, but there's also great potential for misuse. For example, most people would agree that if a==b is true, and b==c is also true, then a==c should be true too, but because of the data type conversion rules that JS applies for the == operator, you have the following:
""==0 // true
0=="0" // true
""=="0" // false!?
Another example follows; what does this very simple function return?
function mystery() {
return
{
something: true
}
}
If you answered an object, you would have been bitten by a missing semicolon. This code is actually interpreted by JS as follows:
function mystery() {
return ;
{
something: true;
}
}
Note the semicolon after return. This function returns undefined, and something is interpreted as a label for an expression that happens to be true; bad! These kinds of situations are common enough, and even if you know what you are doing, getting at least a warning about possible problems with your code could help root out a bug, and that's the kind of warning that ESLint produces.