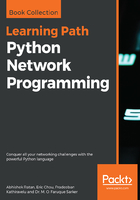
Readability of a program
This is one of the most important aspects of writing a good program. A program needs to be written in such a way that even a layman or a first-time reader of the program should be able to interpret the basics of what is happening.
Variables need to be declared properly so that each variable makes it clear what it stores:
x="India"
y="France"
could have been written better like this:
asiancountry="India"
europecountry="France"
Here's another example:
x=5
y=2
It could be written like this:
biggernumber=5
smallernumber=2
As we can see in the preceding example, if we write a two- or three-line program, we can easily declare the variables in a random way, but things become much more complex, even for a programmer writing their own program, when these random variables are used in a longer program. Just imagine if you have declared the variables as a, b, c, and so on, and later, after using even 10 or 15 more variables, you need to go back to each line of the program to understand what value was declared in a, b, or c.
Another aspect of writing a good program is commenting. Different scripting languages provide different ways of commenting a program. Comments are necessary to ensure we break the flow of each program into sections, with each section having a comment explaining the use of that section. A very good example is if you declare a function. A function named Cooking, for example, and another function named CookingPractice might sound confusing because of their names. Now, if we add a comment to the Cooking method saying this function is to master the art of cooking when you have learned how to cook, and add a comment to CookingPractice saying this method is to learn cooking, this can make things very easy for someone reading through the program.
A programmer now can easily interpret that whenever he wants to learn to cook, he has to call CookingPractice and not the Cooking method. Comments don't have any special meaning in any programming language, and they are ignored when the machine is trying to convert the programming language to machine instructions. Hence, comments are only for programmers and to make readers aware of what is happening in a program. A comment should also be placed with every complex condition, loop, and so on, to clarify the usage of that specific condition or loop.