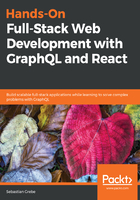
Logging in Node.js
The most popular logging package for Node.js is called winston. Configure winston by following the steps below:
- Install winston with npm:
npm install --save winston
- We create a new folder for all of the helper functions of the back end:
mkdir src/server/helpers
- Then, insert a logger.js file in the new folder with the following content:
import winston from 'winston';
let transports = [
new winston.transports.File({
filename: 'error.log',
level: 'error',
}),
new winston.transports.File({
filename: 'combined.log',
level: 'verbose',
}),
];
if (process.env.NODE_ENV !== 'production') {
transports.push(new winston.transports.Console());
}
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
transports,
});
export default logger;
This file can be imported everywhere where we want to log.
In the preceding code, we defined the standard transports for winston. A transport is nothing more than the way in which winston separates and saves various log types in different files.
The first transport generates an error.log file where only real errors are saved.
The second transport is a combined log where we save all other log messages, such as warnings or info logs.
If we are running the server in a development environment, which we are currently doing, we add a third transport. We will also directly log all messages to the console while developing on the server.
Most people who are used to JavaScript development know the difficulty with console.log. By directly using winston, we can see all messages in the terminal, but we do not need to clean the code from console.log either, as long as the things we log make sense, of course.
To test this, we can try the winston logger in the only mutation we have.
In resolvers.js, add this to the top of the file:
import logger from '../../helpers/logger';
Now, we can extend the addPost function by logging the following:
logger.log({ level: 'info', message: 'Post was created' });
When you send the mutation now, you will see that the message was logged to the console.
Furthermore, if you look in the root folder of your project, you will see the error.log and combined.log files. The combined.log file should contain the log from the console.
Now that we can log all operations on the server, we should explore Postman to send requests comfortably.