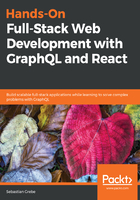
CORS in Express.js
We want our GraphQL API to be accessible from any website, app, or system. A good idea might be to build an app or offer the API to other companies or developers so that they can use it. When using APIs via Ajax, the main problem is that the API needs to send the correct Access-Control-Allow-Origin header.
For example, if you build the API, publicize it under https://api.example.com, and try to access it from https://example.com without setting the correct header, it won't work. The API would need to set at least example.com inside the Access-Control-Allow-Origin header to allow this domain to access its resources. It seems a bit tedious, but it makes your API open to cross-site requests which you should always be aware of.
Allow CORS (Cross-origin resource sharing) requests with the following command to the index.js file:
app.use(cors());
This command handles all of the problems we usually have with cross-origin requests at once. It merely sets a wildcard with * inside of Access-Control-Allow-Origin, allowing anyone from anywhere to use your API, at least in the first instance. You can always secure your API by offering API keys or by only allowing access to logged-in users. Enabling CORS only allows the requesting site to receive the response.
Furthermore, the command also implements the OPTIONS route for the whole application.
The OPTIONS method or request is made every time we use Cross-origin resource sharing. This action is what's called a preflight request, which ensures that the responding server trusts you. If the server does not respond correctly to the OPTIONS preflight, the actual method, such as POST, will not be made by the browser at all.
Our application is now ready to serve all routes appropriately and respond with the right headers.
We can move on now and finally set up a GraphQL server.