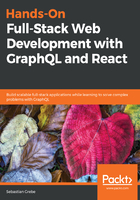
Render your first React component
There are many best practices for React. The central philosophy behind it is to split up our code into separate components where possible. We are going to cover this approach in more detail later in Chapter 5, Reusable React Components.
Our index.js file is the main starting point of our front end code, and this is how it should stay. Do not include any business logic in this file. Instead, keep it as clean and slim as possible.
The index.js file should include this code:
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
ReactDOM.render(<App/>, document.getElementById('root'));
The release of ECMAScript 2015 introduced the import feature. We use it to require our npm packages, react and react-dom , and our first custom React component, which we must write now.
Of course, it is essential for us to cover the sample Hello World program.
Create the App.js file next to your index.js file, with the following content:
import React, { Component } from 'react';
export default class App extends Component {
render() {
return (
<div>Hello World!</div>
)
}
}
This class is exported and then imported by the index.js file. As explained before, we are now actively using ReactDOM.render in our index.js file.
The first parameter of ReactDOM.render is the component that we want to render, which is the App class displaying the Hello World! message. The second parameter is the browser's DOMNode, where it should render. We receive DOMNode with plain document.getElementById JavaScript.
We defined our root element when we created the index.html file before. After saving the App.js file, webpack will try to build everything again. However, it shouldn't be able to do that. Webpack will encounter a problem bundling our index.js file because of the <App /> tag syntax we are using in the ReactDOM.render method. It was not transpiled to a normal JavaScript function.
We configured webpack to load Babel for our JS file but did not tell Babel what to transpile and what not to transpile.
Let's create a .babelrc file in the root folder with this content:
{
"plugins": [
["@babel/plugin-proposal-decorators", { "legacy": true }],
"@babel/plugin-proposal-function-sent",
"@babel/plugin-proposal-export-namespace-from",
"@babel/plugin-proposal-numeric-separator",
"@babel/plugin-proposal-throw-expressions",
["@babel/plugin-proposal-class-properties", { "loose": false }]
],
"presets": ["@babel/env","@babel/react"]
}
Here, we told Babel to use @babel/preset-env and @babel/preset-react, installed together with webpack. These presets allow Babel to transform specific syntax such as JSX, which we use to create normal JavaScript that all browsers can understand and that webpack is able to bundle. Furthermore, we are using some Babel plugins we installed too, because they transform specific syntax not covered by the presets.