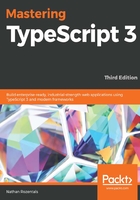
Null and undefined
In JavaScript, if a variable has been declared, but not assigned a value, then querying its value will return undefined. JavaScript also includes the keyword null, in order to distinguish between cases where a variable is known, but has no value (null), and where it has not been defined in the current scope (undefined). Consider the following JavaScript code:
function testUndef(test) { console.log('test parameter :' + test); } testUndef(); testUndef(null);
Here, we have defined a function named testUndef, that takes a single argument named test. Within this function, we are simply logging the value to the console. We then call it in two different ways.
The first call to the testUndef function does not have any arguments. This is, in effect, calling the function without knowing, or without caring, what arguments it needs. Remember that arguments to JavaScript functions are optional. In this case, the value of the test argument within the testUndef function will be undefined, and the output will be as follows:
test parameter :undefined
The second call to the testUndef function passes null as the first argument. This is basically saying that we are aware that the function needs an argument, but we choose to call it without a value. The output of this function call will be as follows:
test parameter :null
TypeScript has included two keywords that we can use in these cases, named null and undefined. Let's rewrite this function in TypeScript, as follows:
function testUndef(test : null | number) { console.log('test parameter :' + test); }
Here, we have defined the testUndef function to allow for the function to be called with either a number value, or a null value. If we try to call this function in TypeScript without any arguments, as we did in JavaScript:
testUndef();
TypeScript will generate an error:
error TS2554: Expected 1 arguments, but got 0.
Clearly, the TypeScript compiler is ensuring that we call the testUndef function with either a number, or null. It will not allow us to call it without any arguments.
This ability to specify that a function can be called with a null value allows us to ensure that the correct use of our function is known at compile time.
Similarly, we can define an object to allow undefined values, as follows:
let x : number | undefined; x = 1; x = undefined; x = null;
Here, we have defined a variable named x, that is allowed to hold either a number, or undefined. We then attempt to assign the values 1, undefined, and null to this variable. Compiling this code will result in the following TypeScript error:
error TS2322: Type 'null' is not assignable to type 'number | undefined'.
The TypeScript compiler, therefore, is protecting our code to ensure that the variable x can only hold either a number or undefined, while not allowing it to hold a null value.