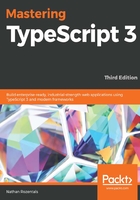
Rest parameters
The JavaScript language also allows a function to be called with a variable number of arguments. To illustrate this point, we can make use of a quirk of the JavaScript language. Every JavaScript function has access to a special variable, named arguments, that can be used to retrieve all arguments that have been passed into the function. As an example of this, consider the following JavaScript code:
function testArguments() { if (arguments.length > 0) { for (var i = 0; i < arguments.length; i++ ) { console.log("argument[" + i + "] = " + arguments[i]); } } } testArguments(1,2,3); testArguments("firstArg");
Here, we have defined a function, named testArguments, which does not have any named parameters. Note, though, that we can use the special variable, named arguments, to test whether the function was called with any arguments. In our sample, we simply loop through the arguments array, and log the value of each argument to the console, by using an array index, arguments[i]. The output of this code is as follows:
argument[0] = 1 argument[1] = 2 argument[2] = 3 argument[0] = firstArg
In order to express the equivalent function definition in TypeScript, we will need to use a syntax that is known as rest parameter syntax. Rest parameters use the TypeScript syntax of three dots (...) in the function declaration to express a variable number of function parameters. Here is the equivalent testArguments function, expressed in TypeScript:
function testArguments(... argArray: number []) { if (argArray.length > 0) { for (var i = 0; i < argArray.length; i++) { console.log(`argArray[${i}] = ${argArray[i]}`); // use JavaScript arguments variable console.log(`arguments[${i}] = ${arguments[i]}`) } } } testArguments(9); testArguments(1,2,3);
Note the use of the ...argArray: number[] syntax for our testArguments function parameter. This syntax is telling the TypeScript compiler that the function can accept any number of arguments, as long as each argument is a number. We can therefore call this function as seen in the last two lines of the preceding code with any number of numeric values. There are also two console.log statements in this for loop. The first uses argArray[i], which is referencing the rest parameter name, and the second uses the standard JavaScript arguments variable, arguments[i], which is still available for use in TypeScript.
The output of this code is as follows:
argArray[0] = 9 arguments[0] = 9 argArray[0] = 1 arguments[0] = 1 argArray[1] = 2 arguments[1] = 2 argArray[2] = 3 arguments[2] = 3
We can also combine normal parameters along with rest parameters in a function definition, as long as the rest parameters are the last to be defined in the parameter list, as follows:
function testNormalAndRestArguments( arg1: string, arg2, number, ...argArray: number[] ) { }
Here, we have two normal parameters named arg1 and arg2, and then an argArray rest parameter. Mistakenly placing the rest parameter at the beginning of the parameter list will generate a compile error.