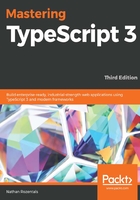
Dotted property types
When working with JavaScript objects, it is fairly common to define them with property names that are strings, as can be seen in the following code:
let normalObject = { id : 1, name : "test" } let stringObject = { "testProperty": 1, "anotherProperty": "this is a string" }
Here, we have defined an object named normalObject that has an id and a name property. We can access these properties by referencing normalObject.id, or normalObject.name, as we have seen many times before. Note, however, the minor change in the definition of the stringObject object. Here, each property name is enclosed in double quotes ( "" ), making it a string property. We can reference these string properties in two ways:
let testProperty = stringObject.testProperty; console.log(`testPropertyValue = ${testProperty}`); let testStringProperty = stringObject["testProperty"]; console.log(`"testPropertyValue" = ${testStringProperty}`);
Here, we are using both the stringObject.testProperty syntax, as well as the stringObject["testProperty"] syntax to access the testProperty property. To TypeScript, these are one and the same, as the output of this code snippet shows:
testPropertyValue = 1 "testPropertyValue" = 1