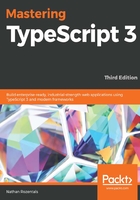
Strong typing
JavaScript is not strongly typed. It is a language that is very dynamic, as it allows objects to change their properties and behavior on the fly. As an example of this, consider the following code:
var test = "this is a string"; console.log('test=' + test); test = 1; console.log('test=' + test); test = function (a, b) { return a + b; } console.log('test=' + test);
On the first line of this code snippet, a variable named test is declared, and assigned a string value. To ensure that this is the case, we have logged the value to the console. We then assign a numeric value to the test variable, and again log its value to the console. Note, however the final snippet of code. We are assigning a function that takes two parameters to the test variable. If we run this code, we will get the following results:
test = this is a string
test = 1
test = function (a, b) {
return a + b;
}
Here, we can clearly see the changes we are making to the test variable. It changes from a string value to a numeric value, and then becomes a function.
Changing the type of a variable at runtime can be a very dangerous thing to do, and can cause untold problems. This is why traditional object-oriented languages enforce strict typing. In other words, they do not allow the nature of a variable to change once declared.
While all of the preceding code is valid JavaScript – and could be justified – it is quite easy to see how this could cause runtime errors during execution. Imagine that you were responsible for writing a library function to add two numbers, and then another developer inadvertently reassigned your function to subtract these numbers instead.
These sorts of errors may be easy to spot in a few lines of code, but it becomes increasingly difficult to find and fix these as your code base and development team expands.