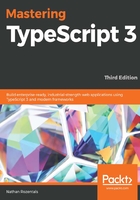
Static properties
Similar to static functions, classes can also have static properties. If a property of a class is marked as static, then each instance of this class will have the same value for the property. In other words, all instances of the same class will share the static property. Consider the following code:
class StaticProperty { static count = 0; updateCount() { StaticProperty.count ++; } }
This class definition uses the static keyword on the class property count. It has a single function named updateCount, which increments the static count property. Note the syntax within the updateCount function. Normally, we would use the this keyword to access properties of a class.
Here, however, we need to reference the full name of the property, including the class name, that is, StaticProperty.count to access this property, and is similar to what we have seen for static functions. We can then use this class, as follows:
let firstInstance = new StaticProperty(); console.log(`StaticProperty.count = ${StaticProperty.count}`); firstInstance.updateCount(); console.log(`StaticProperty.count = ${StaticProperty.count}`); let secondInstance = new StaticProperty(); secondInstance.updateCount(); console.log(`StaticProperty.count = ${StaticProperty.count}`);
This code snippet starts with a new instance of the StaticProperty class named firstInstance. We are then logging the value of StaticProperty.count to the console. We then call the updateCount function on this instance of the class, and again log the value of StaticProperty.count to the console. We then create another instance of this class, named secondInstance, call the updateCount function, and log the value of StaticProperty.count to the console. This code snippet will output the following:
StaticProperty.count = 0 StaticProperty.count = 1 StaticProperty.count = 2
What this output shows us is that the static property named StaticCount.count is indeed shared between two instances of the same class, that is, firstInstance and secondInstance. It starts out as 0, and is incremented when firstInstance.updateCount is called. When we create a second instance of the class, it also retains its original value of 1 for this class instance. When secondInstance updates this count, it will then also be updated for firstInstance.