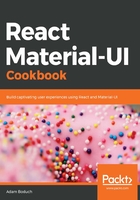
There's more...
You can add a value property to the TabsContainer component so that you can set whichever tab to activate initially. For example, you might want the second tab to be active instead of the first tab when the screen first loads. To do this, you'll have to add a default property value for value, call setValue() if the value state hasn't been set yet, and remove value from the initial state:
function TabContainer({ children, value: valueProp }) {
const [value, setValue] = useState();
const onChange = (e, value) => {
setValue(value);
};
if (value === undefined) {
setValue(valueProp);
}
return (
<Fragment>
<Tabs value={value} onChange={onChange}>
{Children.map(children, child => (
<Tab label={child.props.label} />
))}
</Tabs>
{Children.map(children, (child, index) =>
index === value ? child : null
)}
</Fragment>
);
}
TabContainer.defaultProps = {
value: 0
};
The default property is necessary because the value state is now undefined by default. The setValue() method is called if the value state is undefined. If it is, then you can set it by passing it the value property value.
Now, you can pass this property to your component to change the initially-active tab:
<TabContainer value={1}>
<TabContent label="Item One">Item One Content</TabContent>
<TabContent label="Item Two">Item Two Content</TabContent>
<TabContent label="Item Three">Item Three Content</TabContent>
</TabContainer>
The value property is set to 1. It's a zero-based index, which means that the second tab will be active by default:

When the user starts clicking on other tabs, the value state updates as expected—only the initially-active tab is impacted by this change.