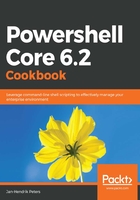
上QQ阅读APP看书,第一时间看更新
How to do it...
Install and start PowerShell Core and execute the following steps:
- In order to be able to develop your own provider, you need to install SHiPS from the gallery first. Alternatively, you can follow the steps outlined at https://github.com/powershell/ships to compile it yourself with .NET Core:
# Install SHiPS
Install-Module -Name SHiPS -Force -Scope CurrentUser
- SHiPS is based on PowerShell classes. First of all, you might want to add your root container:
# SHiPS providers are based around PowerShell classes.
# To create a new directory, or container, your class needs to
# inherit from the class ShipsDirectory
class MyContainerType : Microsoft.PowerShell.SHiPS.SHiPSDirectory
{
# Your new container should now implement the function GetChildItem() at the very least.
# In order to actually return child items, your container needs content!
MyContainerType([string]$name): base($name)
{
}
[object[]] GetChildItem()
{
$obj = @()
$obj += [MyFirstLeafType]::new();
return $obj;
}
}
- Add another container that is nested in your root, as well as one or two leafs:
# Leafs are the child items of your containers that can't contain any more items themselves.
# Containers are still allowed to contain more containers though.
class MyFirstContainerType : Microsoft.PowerShell.SHiPS.SHiPSDirectory
{
MyFirstContainerType([string]$name): base($name)
{
}
}
class MyFirstLeafType : Microsoft.PowerShell.SHiPS.SHiPSLeaf
{
MyFirstLeafType () : base ("MyFirstLeafType")
{
}
[string]$LeafProperty = 'Value'
[int]$LeafLength = 42
[datetime]$LeafDate = $(Get-Date)
}
- Lastly, store your provider definition as a module and import it:
# Take a look at the sample code
psedit .\ShipsProvider.psm1
# In order to mount your provider drive, you can simply use New-PSDrive
Import-Module -Name .\ShipsProvider -Force
psedit is a handy function available in Visual Studio Code to open files in the editor! If you are working in another IDE, you might need a different command to view the code. Try Get-Content instead.
- With SHiPS and your module imported, you can mount your drive. Notice that the root of your drive relates to the root class that contains your hierarchy:
New-PSDrive -Name MyOwnDrive -PSProvider SHiPS -Root "ShipsProvider#MyContainerType"
- Examine it with the item cmdlets:
# Now you can use the item cmdlets at your leisure
Get-ChildItem -Path MyOwnDrive:\MyFirstContainerType
Get-ChildItem -Path MyOwnDrive: -Recurse
- Notice how your leafs and containers are displayed, as follows:
