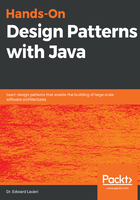
上QQ阅读APP看书,第一时间看更新
Programming the design pattern
The first part of our source code includes import statements for ArrayList and Iterator. In the main() method, we create a colonies ArrayList of strings. We then populate 12 elements to the ArrayList:
import java.util.ArrayList;
import java.util.Iterator;
public class IteratorExample {
public static void main(String[] args) {
ArrayList<String> colonies = new ArrayList<>();
colonies.add("Aerlion");
colonies.add("Aquaria");
colonies.add("Canceron");
colonies.add("Caprica");
colonies.add("Gemenon");
colonies.add("Leonis");
colonies.add("Libran");
colonies.add("Picon");
colonies.add("Sagittaron");
colonies.add("Scorpia");
colonies.add("Tauron");
colonies.add("Virgon");
The second half of the source code does three things. First, it instantiates an iterator named myIterator. Next, a simple text header is printed to the console. Lastly, the code iterates through the ArrayList, printing each element:
// instantiate iterator
Iterator myIterator = colonies.iterator();
// console output
System.out.println("\n\nOriginal Colonies of Kobol:");
// iterate through the list
while (myIterator.hasNext())
System.out.println("\t\t" + myIterator.next());
}
}
The iteration was able to take place without knowing that the colonies object stored its data in an ArrayList.
The output of our sample application is illustrated here:

Iterator application output
This section featured the source code and output, demonstrating the iterator design pattern.