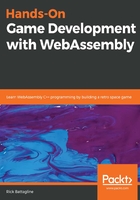
Initializing SDL
Like in other targets for C/C++, the code begins execution from within the main function. We are going to start our main function by declaring some variables:
int main() {
SDL_Window *window;
SDL_Renderer *renderer;
SDL_Rect dest = {.x = 160, .y = 100, .w = 0, .h = 0 };
TTF_Font *font;
SDL_Texture *texture;
The first two variables are the SDL_Window and SDL_Renderer objects. The window object would define the application window that we would be rendering into if we were writing code for a Windows, Mac, or Linux system. When we build for WebAssembly, there is a canvas in our HTML, but SDL still requires a window object pointer for initialization and cleanup. All calls to SDL use the renderer object to render images to the canvas.
The SDL_Rect dest variable is a rectangle that represents the destination where we will be rendering onto the canvas. We will render to the center of the 320x200 canvas, so we will start with an x and y value of 160 and 100. We do not yet know the width and height of the text we will render, so, at this point, we are going to set w and h to 0. We will reset this value later, so, in theory, we could set it to anything.
The TTF_Font *font variable is a pointer to the SDL_TTF library's font object. Later, we will use that object to load up a font from the virtual filesystem and render that font to the SDL_Texture *texture pointer variable. The SDL_Texture variables are used by SDL to render sprites to the canvas.
These next few lines are used to do some initialization work in SDL:
SDL_Init( SDL_INIT_VIDEO );
TTF_Init();
SDL_CreateWindowAndRenderer( 320, 200, 0, &window, &renderer );
The SDL_Init function is called with a single flag initializing only the video subsystem. As a side note, I am not aware of any use case for SDL that does not require the video subsystem to be initialized. Many developers use SDL as an OpenGL/WebGL graphics rendering system; so, unless you have designed a game that is audio only, you should always pass in the SDL_INIT_VIDEO flag. If you would like to initialize additional SDL subsystems, you would pass in the flags for those subsystems using a Boolean or | operator, as shown in the following code snippet:
SDL_Init( SDL_INIT_VIDEO | SDL_INIT_AUDIO | SDL_INIT_HAPTIC );
If we use the preceding line, SDL would have also initialized the audio and haptic subsystems, but we do not need them right now, so we will not be making that change.
The TTF_Init(); function initializes our TrueType fonts, and SDL_CreateWindowAndRenderer returns a window and renderer object to us. We are passing 320 for the width of the canvas and 200 for the height. The third variable is the window flags. We pass 0 in for that parameter to indicate that we do not need any window flags. Because we are working with the SDL Emscripten port, we do not have control of the window, so these flags do not apply.