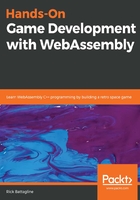
The MoveShip function
We are going to need to jump back into the WebAssembly C module. The webgl.c file is a copied version of canvas.c where the only changes we need to make are inside of the MoveShip function. Here is the new version of MoveShip:
void MoveShip() {
ship_x += 0.002;
ship_y += 0.001;
if( ship_x >= 1.16 ) {
ship_x = -1.16;
}
if( ship_y >= 1.21 ) {
ship_y = -1.21;
}
EM_ASM( ShipPosition($0, $1), ship_x, ship_y );
}
The changes are all conversions from pixel space into WebGL clip space. In the 2D canvas version, we were adding two pixels to the ship's x coordinate and one pixel to the ship's y coordinate every frame. But in WebGL, moving the x coordinate by two would be moving it by the entire width of the screen. So, instead, we have to modify these values into small units that would work with the WebGL coordinate system:
ship_x += 0.002;
ship_y += 0.001;
Adding 0.002 to the x coordinate moves the ship by 1/500th of the width of the canvas each frame. Moving the y coordinate by 0.001 moves the ship on the y-axis by 1/1,000th of the height of the screen each frame. You may notice that in the 2D canvas version of this app, the ship was moving to the right and down. That was because increasing the y coordinate in the 2D canvas coordinate system moves an image down the screen. In the WebGL coordinate system, the ship moves up. The only other thing we have to do is change the coordinates at which the ship wrapped its x and y coordinates to WebGL clip space:
if( ship_x >= 1.16 ) {
ship_x = -1.16;
}
if( ship_y >= 1.21 ) {
ship_y = -1.21;
}
Now that we have all of our source code, go ahead and run emcc to compile our new webgl.html file:
emcc webgl.c -o webgl.html --shell-file webgl_shell.html
Once you have webgl.html compiled, load it into a web browser. It should look like this:

Now that we have all of this working in WebGL, in the next chapter, I will talk about how much easier all of this would have been if we just did it using SDL in the first place.