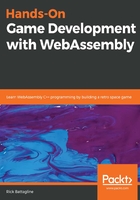
Vertex and UV data
Before we look at a large chunk of scary WebGL JavaScript code, I want to briefly discuss data buffers and how we are going to pass the geometry and texture coordinate data into the shaders. We will be passing in 32-bit floating point data in a large buffer that will contain a combination of the X and Y coordinates for the vertex and UV texture coordinates for that same vertex. UV mapping is the method by which your GPU maps 2D texture coordinates onto 3D geometry:

WebGL and OpenGL accomplish this by assigning a U and V coordinate to every vertex. A UV coordinate of (0,0) assigned to a vertex means that the vertex will be colored based on the color in the texture in the top left corner. A UV coordinate of (1,1) would imply that it would be painted based on what color is in the texture on the bottom right. As we interpolate between the points in our 3D object, we also interpolate between the different UV coordinates inside of the texture. Those UV coordinates can be sampled in our fragment shader using the texture2D built-in function by passing in the texture and the current UV coordinates.
Let's take a look at the vertex and texture data array that we are using inside of this WebGL app:
var vertex_texture_data = new Float32Array([
// X, Y, U, V
0.16, 0.213, 1.0, 1.0,
-0.16, 0.213, 0.0, 1.0,
0.16, -0.213, 1.0, 0.0,
-0.16, -0.213, 0.0, 0.0,
-0.16, 0.213, 0.0, 1.0,
0.16, -0.213, 1.0, 0.0
]);
This data has been typed out in rows and columns. Even though this is a linear array of data, the formatting allows you to see that we have four floating-point values that will be passed in for each vertex. There is a comment above the data showing what each column represents. The first two data values are the X and Y coordinates of the geometry. The second two values are the U and V coordinates that map the texture to the X and Y coordinates in the geometry. There are six rows here, even though we are rendering a rectangle. The reason we need six points instead of just four is that the geometry used by WebGL typically consists of triangles. Because of this, we will need to repeat two of the vertices.