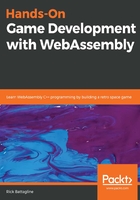
Using Emscripten
We run Emscripten from the command line; therefore, you can use any text editor you choose to write your C/C++ code. Personally, I am partial to Visual Studio Code, which you can download here: https://code.visualstudio.com/download.
One beautiful thing about Visual Studio Code is that it has a built-in command-line terminal, which lets you compile your code without switching windows. It also has an excellent C/C++ extension that you can install. Just search for C/C++ from the extensions menu and install the Microsoft C/C++ Intellisense extension.
Whatever you choose for your text editor or integrated development environment, you need a simple piece of C code to test out the emcc compiler.
- Create a new text file and name it hello.c.
- Type the following code into hello.c:
#include <emscripten.h> #include <stdlib.h>
#include <stdio.h>
int main() { printf("hello wasm\n"); }
- Now I can compile the hello.c file into WebAssembly and generate a hello.html file:
emcc hello.c --emrun -o hello.html
- The --emrun flag is necessary if you want to run the HTML page from emrun. This flag adds code that will capture stdout, stderr, and exit in the C code and emrun will not work without it:
emrun --browser firefox hello.html
Running emrun with the --browser flag will pick the browser where you would like to run the script. The behavior of emrun seems to be different between browsers. Chrome will close the window when the C program exits. That can be annoying because we are just trying to display a simple print message. If you have Firefox, I would suggest running emrun using the --browser flag.
To find out what browsers are available to you, run the following:
emrun --list_browsers
emrun should open an Emscripten-templated HTML file in a browser.
Make sure you have a browser capable of running WebAssembly. The following versions of the major browsers should work with WebAssembly:
- Edge 16
- Firefox 52
- Chrome 57
- Safari 11
- Opera 44