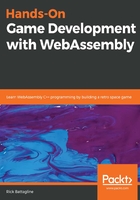
Pointers in memory
WebAssembly's memory model piggybacks on the asm.js memory model, which uses a large typed ArrayBuffer to hold all of the raw bytes to be manipulated by the module. A JavaScript call to WebAssembly.Memory sets up the module's memory buffer in 64 KB pages.
A WebAssembly module can only access data from within this ArrayBuffer. That prevents malicious attacks from WebAssembly that create a pointer to a memory address outside the browser's sandbox. Because of this design, WebAssembly's memory model is just as safe as JavaScript.
In the next section, we will be using C++ pointers in our collider object. If you are a JavaScript developer, you may not be familiar with pointers. A pointer is a variable that holds a memory location instead of the value directly. Let's look at a little bit of code:
int VAR1 = 1;
int* POINTER = &VAR1;
In this code, we have created a VAR1 variable and given it a value of 1. In the second line, we use int* to create a pointer called POINTER. We then initialize that pointer to the address of VAR1 using the & character, which, in C++, is known as the address of operator. This operator gives us the address of the VAR1 that we declared earlier. If we then want to change VAR1, we can do so using the pointer instead of directly, as shown here:
*POINTER = 2;
printf("VAR1=%d\n", VAR1); // prints out "VAR1=2"
Putting the * in front of POINTER tells C++ to set the value in the memory address where POINTER is pointing; * when used in this way is called the dereference operator.
If you would like to learn more about pointers in C++ and how they work, the following article goes into a good deal of detail on the subject: http://www.cplusplus.com/doc/tutorial/pointers/.
In the next section, we will implement compound circle colliders for collision detection on our spaceships.