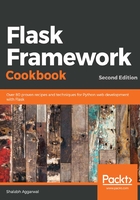
How to do it...
Let's demonstrate this with a small application. This application is very similar to the one we developed in Chapter 1, Flask Configurations.
The first thing to do is to add a new folder named templates under my_app. The application structure should look like the following directory structure:
flask_app/ - run.py my_app/ - __init__.py - hello/ - __init__.py - views.py - templates
We now need to make some changes to the application. The hello_world method in the views file, my_app/hello/views.py, should look like the following lines of code:
from flask import render_template, request
@hello.route('/') @hello.route('/hello') def hello_world(): user = request.args.get('user', 'Shalabh') return render_template('index.html', user=user)
In the preceding method, we look for a URL query argument, user. If it is found, we use it, and if not, we use the default argument, Shalabh. Then, this value is passed to the context of the template to be rendered, that is, index.html, and the resulting template is rendered.
The my_app/templates/index.html template can simply be the following:
<html> <head> <title>Flask Framework Cookbook</title> </head> <body> <h1>Hello {{ user }}!</h1> <p>Welcome to the world of Flask!</p> </body> </html>