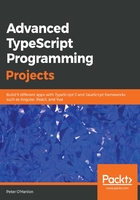
Displaying the personal details interface
We are going to create a class called PersonalDetails. This class is going to render out the core of our interface inside the render method. Again, we are using reactstrap to lay out the various parts of the interface. Before we break down the intricacies of what our render method does, let's take a look at what this all looks like:
import * as React from 'react';
import Button from 'reactstrap/lib/Button';
import Col from 'reactstrap/lib/Col';
import Row from 'reactstrap/lib/Row';
export default class PersonalDetails extends React.Component {
public render() {
return (
<Row>
<Col lg="8">
<Row>
<Col><h4 className="mb-3">Personal details</h4></Col>
</Row>
<Row>
<Col><label htmlFor="firstName">First name</label></Col>
<Col><label htmlFor="lastName">Last name</label></Col>
</Row>
<Row>
<Col>
<input type="text" id="firstName" className="form-control" placeholder="First name" />
</Col>
<Col><input type="text" id="lastName" className="form-control" placeholder="Last name" /></Col>
</Row>
... Code omitted for brevity
<Col>
<Col>
<Row>
<Col lg="6"><Button size="lg" color="success">Load</Button></Col>
<Col lg="6"><Button size="lg" color="info">New Person</Button></Col>
</Row>
</Col>
</Col>
</Row>
);
}
}
As you can see, there's a lot going on in this method; however, the vast majority of it is repeated code used to replicate the row and column Bootstrap elements. If we take a look at the layout for the postcode and phoneNumber elements, for instance, we can see that we are laying out two rows with two explicit columns in each. In Bootstrap terms, one of these Col elements is a large size of three and the other one is a large size of four (we will leave it to Bootstrap to factor in the empty column that remains):
<Row>
<Col lg="3"><label htmlFor="postcode">Postal/ZipCode</label></Col>
<Col lg="4"><label htmlFor="phoneNumber">Phone number</label></Col>
</Row>
<Row>
<Col lg="3"><input type="text" id="postcode" className="form-control" /></Col>
<Col lg="4"><input type="text" id="phoneNumber" className="form-control" /></Col>
</Row>
Looking at the label and input elements, we can see that there are two unfamiliar elements. Surely, the correct key in a label is for and we should use class to refer to a CSS class in our input? The reason we have replacement keys here is that for and class are JavaScript keywords. As React allows us to mix the code and markup language inside a render, React has to use different keywords. This means that we use htmlFor to replace for and className to replace class. Going back to when we talked about the virtual DOM, this gives us a major hint that these HTML elements are copies that serve a similar purpose, rather than the elements themselves.