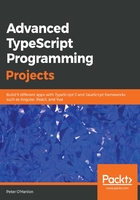
React using tsx components
One question that you might have right now is why does the index file have a different extension? That is, why is it .tsx and not .ts? To answer these questions, we have to change our mental image of the extension slightly and talk about why React uses .jsx files and not .js (the .tsx version is the TypeScript equivalent of .jsx).
These JSX files are extensions of JavaScript that get transpiled to JavaScript. If you were to try and run them as is in JavaScript, then you would get runtime errors if they contained any of these extensions. In traditional React, there is a transpilation phase that takes the JSX file and converts it to JavaScript by expanding out the code to standard JavaScript. Effectively, this is a form of the compilation phase that we get from TypeScript anyway. With TypeScript React, we get the same end result where the TSX file ultimately ends up as a JavaScript file.
So, the question now is why do we actually need these extensions? To answer this, we are going to analyze the index.tsx file. This is what the file looks like with our Bootstrap CSS file added:
import "bootstrap/dist/css/bootstrap.min.css";
import * as React from 'react';
import * as ReactDOM from 'react-dom';
import App from './App';
import registerServiceWorker from './registerServiceWorker';
ReactDOM.render(
<App />,
document.getElementById('root') as HTMLElement
);
registerServiceWorker();
The import statements should be familiar to us by now and registerServiceWorker is the behavior that is added to the code to provide a faster production application by serving assets from a cache, rather than reloading them time and time again. One of the key tenets of React is that it should be as fast as possible and that's where ReactDOM.render comes in. If we read this piece of code, things should become clear. What it is doing is looking for the element marked as root in the HTML page we serve up—we saw this in the index.html file. The reason we use the as HTMLElement syntax here is that we want to let TypeScript know what type this is (this parameter either has to derive from an element or be null—yes, that does mean that underlying this is a union type).
Now, the reason we need a special extension is because of the piece of code that says <App />. What we are doing here is inlining a piece of XML code into our statement. In this particular instance, we are telling our render method to render out a component called App, that has been defined in the App.tsx file.