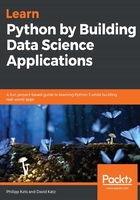
Lists
Lists are probably the most frequently used type of data structure in Python. A list is a simple and ordered 1D array of elements. Each element has its own index number, starting with 0, so the last element always has an index of L-1, where L is the number of elements in the list. Lists can store any mix of data types. They can also store any other data structure. For example, a matrix (2D array) can be represented as a list of lists, or a 3D matrix can be represented as a list of lists of lists. Lists are dynamic, which means you can add or drop items in any number, or even change their order in place, without rebuilding the list from scratch.
You can create a new list by using the list() function, or by using square brackets, with the elements separated by commas:
fruits = ['banana', 'apple', 'plum']
If needed, you can create an empty list as well:
basket = []
another_basket = list()
As with strings, we can check the length of the list by using the built-in len function:
>>> len(fruits)
3
>>> len(basket)
0
We can always add new elements to the end of the list via the .append method:
>>> fruits.append('pineapple')
>>> fruits
['banana', 'apple', 'plum', 'pineapple']
Alternatively, we can insert them into a specific position:
>>> fruits.insert(2, 'orange')
>>> fruits
['banana', 'apple', 'orange', 'plum', 'pineapple']
We can also merge them with another list by using .extend:
>>> fruits.extend(['melon', 'watermelon'])
In some cases, you may want to retrieve one element and also remove it from the list. One such example is if you need to process elements one by one. For that, the .pop() method is ideal, as it will return the element from the end of the list and remove it from the list at the same time. In the following example, we pop (remove) the last value from the list. After that, unsurprisingly, the length of the list is decreased by one:
>>> len(fruits)
7
>>>fruits.pop()
'watermelon'len(fruits)
>>> 6
Finally, you can use the in statement to check whether the list contains a certain element. This will also work with any other iterables:
>>> 'melon' in fruits
True
Now, let's talk about an important property of lists, strings, and many other data structures: slicing.