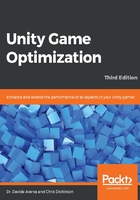
A global messaging system
The final suggested approach to solve the problem of interobject communication is to implement a global messaging system that any object can access and send messages through to any object that may be interested in listening to that specific type of message. Objects can send messages or listen for them (sometimes both!), and the responsibility is on the listener to decide what messages they are interested in. The message sender can broadcast the message without caring at all who is listening, and a message can be sent through the system regardless of the specific contents of the message. This approach is by far the most complex and may require some effort to implement and maintain, but it is an excellent long-term solution to keep our object communication modular, decoupled, and fast as our application gets more and more complex.
The kinds of message we wish to send can take many forms, including data values, references, instructions for listeners, and more, but they should all have a common, basic definition that our messaging system can use to determine what the message is and who it is intended for.
The following is a simple class definition for a Message object:
public class Message {
public string type;
public Message() { type = this.GetType().Name; }
}
The Message class constructor caches the message's type in a local string property to be used later for cataloging and distribution purposes. Caching this value is important, as each call to GetType().Name will result in a new string being allocated, and we've previously learned that we want to minimize this activity as much as possible.
Any custom messages can contain whatever superfluous data they wish so long as they derive from this base class, which will allow it to be sent through our messaging system. Take note that despite acquiring type from the object during its base class constructor, the name property will still contain the name of the derived class, not the base class.
Moving on to our MessagingSystem class, we should define its features by what kind of requirements we need it to fulfill:
- It should be globally accessible
- Any object (MonoBehaviour or not) should be able to register/deregister as listeners to receive specific message types (that is, the observer design pattern)
- Registering objects should provide a method to call when the given message is broadcasted from elsewhere
- The system should send the message to all listeners within a reasonable time frame, but not choke on too many requests at once