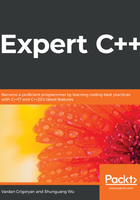
Classes from the compiler perspective
First of all, no matter how monstrous the class from earlier may seem in comparison to the previously introduced struct, the compiler will translate it into the following code (we slightly modified it for the sake of simplicity):
struct Product {
std::string name_;
bool available_;
double price_;
int rating_;
};
// we forced the compiler to generate the default constructor
void Product_constructor(Product&);
void Product_copy_constructor(Product& this, const Product&);
void Product_move_constructor(Product& this, Product&&);
// default implementation
Product& operator=(Product& this, const Product&);
// default implementation
Product& operator=(Product& this, Product&&);
void Product_set_name(const std::string&);
// takes const because the method was declared as const
std::string Product_name(const Product& this);
void Product_set_availability(Product& this, bool b);
bool Product_availability(const Product& this);
std::ostream& operator<<(std::ostream&, const Product&);
std::istream& operator>>(std::istream&, Product&);
Basically, the compiler generates the same code that we introduced earlier as a way to mimic class behavior using a simple struct. Though compilers vary in techniques and methods of implementing the C++ object model, the preceding example is one of the popular approaches practiced by compiler developers. It balances the space and time efficiency in accessing object members (including member functions).
Next, we should consider that the compiler edits our code by augmenting and modifying it. The following code declares the global create_apple() function, which creates and returns a Product object with values specific to an apple. It also declares a book object in the main() function:
Product create_apple() {
Product apple;
apple.set_name("Red apple");
apple.set_price("0.2");
apple.set_rating(5);
apple.set_available(true);
return apple;
}
int main() {
Product red_apple = create_apple();
Product book;
Product* ptr = &book;
ptr->set_name("Alice in Wonderland");
ptr->set_price(6.80);
std::cout << "I'm reading " << book.name()
<< " and I bought an apple for " << red_apple.price()
<< std::endl;
}
We already know that the compiler modifies the class to translate it into a struct and moves member functions to the global scope, each of which takes the reference (or a pointer) to the class as its first parameter. To support those modifications in the client code, it should also modify all access to the objects.
Here's how we will assume the compiler modifies the preceding code (we used the word assume because we're trying to introduce a compiler-abstract rather than a compiler-specific approach):
void create_apple(Product& apple) {
Product_set_name(apple, "Red apple");
Product_set_price(apple, 0.2);
Product_set_rating(apple, 5);
Product_set_available(apple, true);
return;
}
int main() {
Product red_apple;
Product_constructor(red_apple);
create_apple(red_apple);
Product book;
Product* ptr;
Product_constructor(book);
Product_set_name(*ptr, "Alice in Wonderland");
Product_set_price(*ptr, 6.80);
std::ostream os = operator<<(std::cout, "I'm reading ");
os = operator<<(os, Product_name(book));
os = operator<<(os, " and I bought an apple for ");
os = operator<<(os, Product_price(red_apple));
operator<<(os, std::endl);
// destructor calls are skipped because the compiler
// will remove them as empty functions to optimize the code
// Product_destructor(book);
// Product_destructor(red_apple);
}
The compiler also optimized the call to the create_apple() function to avoid temporary object creation. We will discuss the invisible temporaries that were generated by the compiler later in this chapter.