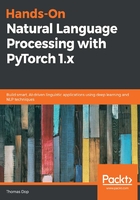
Installing and using PyTorch 1.x
Like most Python packages, PyTorch is very simple to install. There are two main ways of doing so. The first is to simply install it using pip in the command line. Simply type the following command:
pip install torch torchvision
While this installation method is quick, it is recommended to install using Anaconda instead, as this includes all the required dependencies and binaries for PyTorch to run. Furthermore, Anaconda will be required later to enable training models on a GPU using CUDA. PyTorch can be installed through Anaconda by entering the following in the command line:
conda install torch torchvision -c pytorch
To check that PyTorch is working correctly, we can open a Jupyter Notebook and run a few simple commands:
- To define a Tensor in PyTorch, we can do the following:
import torch
x = torch.tensor([1.,2.])
print(x)
This results in the following output:
Figure 2.1 – Tensor output
This shows that tensors within PyTorch are saved as their own data type (not dissimilar to how arrays are saved within NumPy).
- We can perform basic operations such as multiplication using standard Python operators:
x = torch.tensor([1., 2.])
y = torch.tensor([3., 4.])
print(x * y)
This results in the following output:
Figure 2.2 – Tensor multiplication output
- We can also select inpidual elements from a tensor, as follows:
x = torch.tensor([[1., 2.],[5., 3.],[0., 4.]])
print(x[0][1])
This results in the following output:

Figure 2.3 – Tensor selection output
However, note that unlike a NumPy array, selecting an inpidual element from a tensor object returns another tensor. In order to return an inpidual value from a tensor, you can use the .item() function:
print(x[0][1].item())
This results in the following output:

Figure 2.4 – Output of the .item() function
Tensors
Before we continue, it is important that you are fully aware of the properties of a tensor. Tensors have a property known as an order, which essentially determines the dimensionality of a tensor. An order one tensor is a tensor with a single dimension, which is equivalent to a vector or list of numbers. An order 2 tensor is a tensor with two dimensions, equivalent to a matrix, whereas a tensor of order 3 consists of three dimensions. There is no limit to the maximum order a tensor can have within PyTorch:

Figure 2.5 – Tensor matrix
You can check the size of any tensor by typing the following:
x.shape
This results in the following output:

Figure 2.6 – Tensor shape output
This shows that this is a 3x2 tensor (order 2).