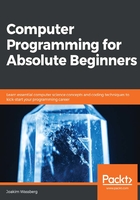
Preface
Welcome to the beautiful world of programming. Programming is an art form in which you will use your imagination and creativity to create things. If you know how to program, your possibilities will be endless.
You can use it to create a fun game. Or maybe you want to automate things in your life. Maybe you want to become a professional programmer, and then you will use your skills to work in teams with others to create solutions that will be used by many people for a long time.
Having programming skills is also something that is needed in more and more professions. Your job title will not be that of a software developer. Instead, programming will be a tool that you can use.
I have been teaching programming for over 30 years. I have been teaching people of all ages, from beginners to senior professional developers. During this time, I have seen patterns, especially among beginners.
It took some time before I realized what made so many of my beginner students struggle with grasping programming. The problem was that they had to learn two things at once. First, they needed to understand the very concepts of programming. There are so many concepts and words, both new ones and some that they already know, but that have a slightly different meaning. Secondly, they will also need to learn a programming language. Taking in all this at the same time will become overwhelming for many people. For some, it will be so much that they give up programming for good.
The idea of this book is to focus on one of the two things you need to learn. This book will not focus on any language, but instead, teach you the concepts you need to know and understand to become a programmer. After you have read this book, you can learn any programming language you want, and when you do, you can focus on just learning the language, as the rest you will already know.
With this book, I also want to put things into context, so there will be a bit of history, and some parts will be rather technical. I believe that if you are going to learn something, you cannot just scratch the surface. You need to pe into it and see how things work.
And yes, I was that kid who disassembled my RC car to see how it worked.
Good luck with your endeavors to learn this fantastic art.
This book was written to the music of Talking Heads, and I suggest you listen to it as you read it.
Who this book is for?
This book is written for everyone who is either just curious about computer programming and wants to know more about the topic, or is about to learn their first programming language and wants a solid introduction to the topic. It doesn't matter whether your goal is to create small hobby applications or whether you want to be well prepared for your university programming courses.
What this book covers?
Chapter 1, Introduction to Computer Programs, will give you an understanding of how computer programs work and how they interact with the computer hardware.
Chapter 2, Introduction to Programming Languages, will teach you the evolution of programming languages, how they are related, introduce different types of languages, and give you a basic understanding of some fundamental programming concepts.
Chapter 3, Types of Applications, explores how software comes in many forms and is created to solve a wide variety of problems. This chapter will introduce you to some of the essential application types and give you an understanding of how they work.
Chapter 4, Software Projects and How We Organize Our Code, covers how, when writing programs that are beyond the most trivial level, we will need to organize our code into several code files. In this chapter, we look at how this can be done efficiently. We will also see how we can incorporate code written by others into our software projects.
Chapter 5, Sequence – The Basic Building Block of a Computer Program, outlines how a computer program is, at the most fundamental level, built by putting statements in the correct sequence. We will see how this is done and how the computer will execute these statement sequences when our programs run.
Chapter 6, Working with Data – Variables, explores how all computer programs will perform operations on data and modify it in some way. In this chapter, we will get to know the types of data we will work with when programming and what types of operations we can perform on them.
Chapter 7, Program Control Structures, discusses how, when writing programs, we need to control the path that the execution takes through our code. To help us, we have different types of control structures that we can use to accomplish this.
Chapter 8, Understanding Functions, explores functions as a fundamental concept in programming that let us package code into a reusable unit. In this chapter, we will see what functions are and how they can be used.
Chapter 9, When Things Go Wrong – Bugs and Exceptions, reminds us that things will not always go according to plan. The code we have written might contain errors, or we might get data that we can't work with. In this chapter, we will see how we can deal with both situations.
Chapter 10, Programming Paradigms, explores several ideas for how we should write and structure our code to be able to write programs as efficiently as possible. These are called paradigms, and we will look at the most prominent ones in this chapter.
Chapter 11, Programming Tools and Methodologies, examines how programmers use different tools when developing software. We will look at some of them in this chapter, and also consider how teams of programmers can collaborate efficiently.
Chapter 12, Code Quality, explores the many aspects of code quality. How can we write programs that run fast, or efficiently use the computer's resources? How can we write code that can easily be read and understood by other programmers that will need to modify our code? These are some of the things we will cover in this chapter.
Appendix A – How to Translate the Pseudocode into Real Code, covers how to translate pseudo code into different programming languages.
Appendix B - Dictionary has all the words which are unique or have a technical meaning and are used in the book.
To get the most out of this book
If you are using the digital version of this book, we advise you to type the code yourself. Doing so will help you avoid any potential errors related to copying/pasting of code.
Download the example code files
You can download the example code files for this book from your account at www.packt.com. If you purchased this book elsewhere, you can visit www.packtpub.com/support and register to have the files emailed directly to you.
You can download the code files by following these steps:
- Log in or register at www.packt.com.
- Select the Support tab.
- Click on Code Downloads.
- Enter the name of the book in the Search box and follow the onscreen instructions.
Once the file is downloaded, please make sure that you unzip or extract the folder using the latest version of:
- WinRAR/7-Zip for Windows
- Zipeg/iZip/UnRarX for Mac
- 7-Zip/PeaZip for Linux
We also have other code bundles from our rich catalog of books and videos available at https://github.com/PacktPublishing/. Check them out!
Download the color images
We also provide a PDF file that has color images of the screenshots/diagrams used in this book. You can download it here: https://static.packt-cdn.com/downloads/9781839216862_ColorImages.pdf.
Conventions used
There are a number of text conventions used throughout this book.
Code in text: Indicates code words in text, database table names, folder names, filenames, file extensions, pathnames, dummy URLs, user input, and Twitter handles. Here is an example: "We know that as this function pides two values, we might get an exception if y is given a value of 0."
A block of code is set as follows:
if current_time > sunset_time {
turn_on_light()
}
Any command-line input or output is written as follows:
SyntaxError: invalid syntax in line 1 column 2
1:2 syntax error: unexpected apple at end of statement
Compilation error (line 1, col 2): Identifier expected
error: unknown: Identifier directly after number (1:2)
Tips or important notes
Appear like this.
Get in touch
Feedback from our readers is always welcome.
General feedback: If you have questions about any aspect of this book, mention the book title in the subject of your message and email us at customercare@packtpub.com.
Errata: Although we have taken every care to ensure the accuracy of our content, mistakes do happen. If you have found a mistake in this book, we would be grateful if you would report this to us. Please visit www.packtpub.com/support/errata, selecting your book, clicking on the Errata Submission Form link, and entering the details.
Piracy: If you come across any illegal copies of our works in any form on the Internet, we would be grateful if you would provide us with the location address or website name. Please contact us at copyright@packt.com with a link to the material.
If you are interested in becoming an author: If there is a topic that you have expertise in and you are interested in either writing or contributing to a book, please visit authors.packtpub.com.
Reviews
Please leave a review. Once you have read and used this book, why not leave a review on the site that you purchased it from? Potential readers can then see and use your unbiased opinion to make purchase decisions, we at Packt can understand what you think about our products, and our authors can see your feedback on their book. Thank you!
For more information about Packt, please visit packt.com.