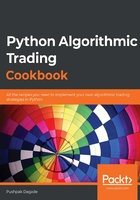
Technical requirements
You will need the following to successfully execute the recipes in this chapter:
- Python 3.7+
- The pyalgotrading Python package ($ pip install pyalgotrading)
The latest Jupyter notebook for this chapter can be found on GitHub at https://github.com/PacktPublishing/Python-Algorithmic-Trading-Cookbook.
The following code will help you set up the broker connection with Zerodha, which will be used in all the recipes in this chapter. Please make sure you have followed these steps before trying out any recipes.
The first thing needed for setting up connectivity with the broker is getting the API keys. The broker would provide unique keys to each customer, typically as an api-key and api-secret key pair. These API keys are chargeable, usually on a monthly subscription basis. You need to get your copy of api-key and api-secret from the broker website before starting this. You can refer to Appendix I for more details.
Execute the following steps:
- Import the necessary modules:
>>> from pyalgotrading.broker.broker_connection_zerodha import BrokerConnectionZerodha
- Get the api_key and api_secret keys from the broker. These are unique to you and will be used by the broker to identify your Demat account:
>>> api_key = "<your-api-key>"
>>> api_secret = "<your-api-secret>"
>>> broker_connection = BrokerConnectionZerodha(api_key, \
api_secret)
You will get the following output:
Installing package kiteconnect via pip...
Please login to this link to generate your request token: https://kite.trade/connect/login?api_key=<your-api-key>&v=3
If you are running this for the first time and kiteconnect is not installed, pyalgotrading will automatically install it for you. The final output of step 2 will be a link. Click on the link and log in with your Zerodha credentials. If the authentication is successful, you will see a link in your browser's address bar similar to this: https://127.0.0.1/?request_token=<alphanumeric-token>&action=login&status=success.
The following is an example:
https://127.0.0.1/?request_token=H06I6Ydv95y23D2Dp7NbigFjKweGwRP7&action=login&status=success
- Copy the alphanumeric-token and paste it in request_token:
>>> request_token = "<your-request-token>"
>>> broker_connection.set_access_token(request_token)
The broker_connection instance is now ready to perform API calls.
The pyalgotrading package supports multiple brokers and provides a connection object class per broker, with the same methods. It abstracts broker APIs behind a unified interface, so you need not worry about the underlying broker API calls and you can use all the recipes in this chapter as is. Only the procedure to set up the broker connection will vary from broker to broker. You can refer to the pyalgotrading documentation for setting up the broker connection if you are not using Zerodha as your broker. For Zerodha users, the previous steps will suffice.