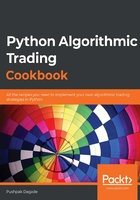
Converting a DataFrame into other formats
This recipe demonstrates the conversion of DataFrame objects into other formats, such as .csv files, json objects, and pickle objects. Conversion into a .csv file makes it easier to further work on the data using a spreadsheet application. The json format is useful for transmitting DataFrame objects over web APIs. The pickle format is useful for transmitting DataFrame objects created in one Python session to another Python session over sockets without having to recreate them.
Getting ready
Make sure the object df is available in your Python namespace. Refer to Creating a pandas.DataFrame object recipe of this chapter to set up this object.
How to do it…
Execute the following steps for this recipe:
- Convert and save df as a CSV file:
>>> df.to_csv('dataframe.csv', index=False)
- Convert df to a JSON string:
>>> df.to_json()
We get the following output:
'{
"timestamp":{
"0":"13-11-2019 09:00:00","1":"13-11-2019 09:15:00",
"2":"13-11-2019 09:30:00","3":"13-11-2019 09:45:00",
"4":"13-11-2019 10:00:00","5":"13-11-2019 10:15:00",
"6":"13-11-2019 10:30:00","7":"13-11-2019 10:45:00",
"8":"13-11-2019 11:00:00","9":"13-11-2019 11:15:00"},
"open":{
"0":71.8075,"1":71.7925,"2":71.7925, "3":71.76,
"4":71.7425,"5":71.775,"6":71.815, "7":71.775,
"8":71.7525,"9":71.7625},
"high"{
"0":71.845,"1":71.8,"2":71.8125,"3":71.765,
"4":71.78,"5":71.8225,"6":71.83,"7":71.7875,
"8":71.7825,"9":71.7925},
"low":{
"0":71.7775,"1":71.78,"2":71.76,"3":71.735,
"4":71.7425,"5":71.77,"6":71.7775,"7":71.7475,
"8":71.7475,"9":71.76},
"close":{
"0":71.7925,"1":71.7925,"2":71.7625,"3":71.7425,
"4":71.7775,"5":71.815,"6":71.78,"7":71.7525,
"8":71.7625,"9":71.7875},
"volume":{
"0":219512,"1":59252,"2":57187,"3":43048,
"4":45863,"5":42460,"6":62403,"7":34090,
"8":39320,"9":20190}}'
- Pickle df to a file:
>>> df.to_pickle('df.pickle')
How it works...
In step 1, you use the to_csv() method to save df as a .csv file. You pass dataframe.csv, a file path where the .csv file should be generated, as the first argument and index as False as the second argument. Passing index as False prevents the index from being dumped to the .csv file. If you want to save the DataFrame along with its index, you can pass the index as True to the to_csv() method.
In step 2, you use the to_json() method to convert df into a JSON string. You do not pass any additional arguments to the to_json() method.
In step 3, you use the to_pickle() method to pickle (serialize) the object. Again you do not pass any additional arguments to the to_pickle() method.
The methods to_csv(), to_json(), and to_pickle() can take more optional arguments than the ones shown in this recipe. Refer to the official docs for complete information on these methods:
- to_csv(): https://pandas.pydata.org/pandas-docs/stable/reference/api/pandas.DataFrame.to_csv.html
- to_json(): https://pandas.pydata.org/pandas-docs/stable/reference/api/pandas.DataFrame.to_json.html
- to_pickle(): https://pandas.pydata.org/pandas-docs/stable/reference/api/pandas.DataFrame.to_pickle.html