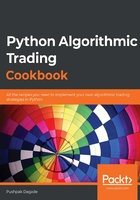
The datetime object and time zones
There are two types of datetime objects—time zone-naive and time zone-aware. Time zone-naive objects do not hold time zone information and timezone-aware objects hold time zone information. This recipe demonstrates multiple time zone related operations on datetime objects: creating time zone-naive and time zone-aware objects, adding time zone information to time zone-aware objects, removing time zone information from time zone-naive objects, and comparing time zone-aware and time zone-naive objects.
How to do it…
Execute the following steps for this recipe:
- Import the necessary modules from the Python standard library:
>>> from datetime import datetime
- Create a time zone-naive datetime object. Assign it to now_tz_naive and print it:
>>> now_tz_unaware = datetime.now()
>>> print(now_tz_unaware)
We get the following output. Your output may differ:
2020-08-12 20:55:50.598800
- Print the time zone information of now_tz_naive. Note the output:
>>> print(now_tz_unaware.tzinfo)
We get the following output:
None
- Create a time zone-aware datetime object. Assign it to now_tz_aware and print it:
>>> now_tz_aware = datetime.now().astimezone()
>>> print(now_tz_aware)
We get the following output. Your output may differ:
2020-08-12 20:55:51.004671+05:30
- Print the time zone information of now_tz_aware. Note the output:
>>> print(now_tz_aware.tzinfo)
We get the following output. Your output may differ:
IST
- Create a new timestamp by adding time zone information to now_tz_naive from now_tz_aware. Assign it to new_tz_aware and print it:
>>> new_tz_aware = now_tz_naive.replace(tzinfo=now_tz_aware.tzinfo)
>>> print(new_tz_aware)
The output is as follows. Your output may differ:
2020-08-12 20:55:50.598800+05:30
- Print the timezone information of new_tz_aware using the tzinfo attribute. Note the output:
>>> print(new_tz_aware.tzinfo)
The output is as follows. Your output may differ:
IST
- Create a new timestamp by removing timezone information from new_tz_aware. Assign it to new_tz_naive and print it:
>>> new_tz_naive = new_tz_aware.replace(tzinfo=None)
>>> print(new_tz_naive)
The output is as follows. Your output may differ:
2020-08-12 20:55:50.598800
- Print the timezone information of new_tz_naive using the tzinfo attribute. Note the output:
>>> print(new_tz_naive.tzinfo)
The output is as follows:
None
How it works...
In step 1, you import the datetime class from the datetime module. In step 2, you create a time zone-naive datetime object using the now() method and assign it to a new attribute now_tz_naive. In step 3, you print the time zone information held by now_tz_naive using the tzinfo attribute. Observe that the output is None as this is a time zone-naive object.
In step 4, you create a time zone-aware datetime object using the now() and astimezone() methods and assign it to a new attribute now_tz_aware. In step 5, you print the time zone information held by now_tz_aware using the tzinfo attribute. Observe that the output is IST and not None; as this is a time zone-aware object.
In step 6, you create a new datetime object by adding time zone information to now_tz_naive. The time zone information is taken from now_tz_aware. You do this using the replace() method (Refer to Modifying datetime objects recipe for more information). You assign this to a new variable, new_tz_aware. In step 7, you print the time zone information held by new_tz_aware. Observe it is the same output as in step 5 as you have taken time zone information from now_tz_aware. Similarly, in step 8 and step 9, you create a new datetime object, new_tz_naive, but this time you remove the time zone information.
There's more
You can use comparison operators only between time zone-naive or time zone-aware datetime objects. You cannot compare a time zone-naive datetime object with a time zone-aware datetime object. Doing so will throw an exception. This is demonstrated in the following steps:
- Compare 2 timezone-naive objects, new_tz_naive and now_tz_naive. Note the output:
>>> new_tz_naive <= now_tz_naive
- Compare 2-time zone-aware objects, new_tz_aware, and now_tz_aware. Note the output:
>>> new_tz_aware <= now_tz_aware
We get the following output:
True
- Compare a time zone-aware object and a time zone-naive object, new_tz_aware, and now_tz_naive. Note the error:
>>> new_tz_aware > now_tz_naive
We get the following output:
-------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-167-a9433bb51293> in <module>
----> 1 new_tz_aware > now_tz_naive
2 # Note: It's expected to get an error below
TypeError: can't compare offset-naive and offset-aware datetimes