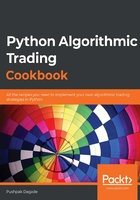
Converting a datetime object to a string
This recipe demonstrates the conversion of the datetime objects into strings which finds application in printing and logging. Also, this is helpful while sending timestamps as JSON data over web APIs.
How to do it…
Execute the following steps for this recipe:
- Import the necessary modules from the Python standard library:
>>> from datetime import datetime
- Fetch the current timestamp along with time zone information. Assign it to now and print it:
>>> now = datetime.now().astimezone()
- Cast now to a string and print it::
>>> print(str(now))
We get the following output. Your output may differ:
2020-08-12 20:55:48.366130+05:30
- Convert now to a string with a specific date-time format using strftime() and print it:
>>> print(now.strftime("%d-%m-%Y %H:%M:%S %Z"))
We get the following output. Your output may differ:
12-08-2020 20:55:48 +0530
How it works...
In step 1, you import the datetime class from the datetime module. In step 2, you fetch the current timestamp with time zone and assign it to a new attribute, now. The now() method of datetime fetches the current timestamp, but without time zone information. Such objects are called time zone-native datetime objects. The astimezone() method adds time zone information from the system local time on this time zone-naive object, essentially converting it to a time zone-aware object. (More information in The datetime object and time zones recipe). In step 3, you cast now to a string object and print it. Observe that the output date format is fixed and may not be of your choice. The datetime module has a strftime() method which can convert the object to a string in a specific format as required. In step 4, you convert now to a string in the format DD-MM-YYYY HH:MM:SS +Z. The directives used in step 4 are described as follows:

A complete list of the directives that can be given to .strptime() can be found at https://docs.python.org/3.7/library/datetime.html#strftime-and-strptime-behavior.