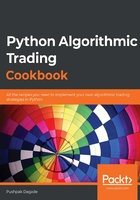
Operations on datetime objects
The datetime and timedelta classes support various mathematical operations to get dates in the future or the past. Using these operations returns another datetime object. . In this recipe, you would create datetime, date, time, and timedelta objects and perform mathematical operations on them.
How to do it…
Follow along with these steps to execute this recipe:
- Import the necessary modules from the Python standard library:
>>> from datetime import datetime, timedelta
- Fetch today's date. Assign it to date_today and print it:
>>> date_today = date.today()
>>> print(f"Today's Date: {date_today}")
We get the following output. Your output may differ:
Today's Date: 2020-08-12
- Add 5 days to today's date using a timedelta object. Assign it to date_5days_later and print it:
>>> date_5days_later = date_today + timedelta(days=5)
>>> print(f"Date 5 days later: {date_5days_later}")
We get the following output. Your output may differ:
Date 5 days later: 2020-08-17
- Subtract 5 days from today's date using a timedelta object. Assign it to date_5days_ago and print it:
>>> date_5days_ago = date_today - timedelta(days=5)
>>> print(f"Date 5 days ago: {date_5days_ago}")
We get the following output. Your output may differ:
Date 5 days ago: 2020-08-07
- Compare date_5days_later with date_5days_ago using the > operator:
>>> date_5days_later > date_5days_ago
We get the following output:
True
- Compare date_5days_later with date_5days_ago using the < operator:
>>> date_5days_later < date_5days_ago
We get the following output:
False
- Compare date_5days_later, date_today and date_5days_ago together using the > operator:
>>> date_5days_later > date_today > date_5days_ago
We get the following output:
True
- Fetch the current timestamp. Assign it to current_timestamp:
>>> current_timestamp = datetime.now()
- Fetch the current time. Assign it to time_now and print it:
>>> time_now = current_timestamp.time()
>>> print(f"Time now: {time_now}")
We get the following output. Your output may differ:
Time now: 20:55:45.239177
- Add 5 minutes to the current time using a timedelta object. Assign it to time_5minutes_later and print it:
>>> time_5minutes_later = (current_timestamp +
timedelta(minutes=5)).time()
>>> print(f"Time 5 minutes later: {time_5minutes_later}")
We get the following output. Your output may differ:
Time 5 minutes later: 21:00:45.239177
- Subtract 5 minutes from the current time using a timedelta object. Assign it to time_5minutes_ago and print it:
>>> time_5minutes_ago = (current_timestamp -
timedelta(minutes=5)).time()
>>> print(f"Time 5 minutes ago: {time_5minutes_ago}")
We get the following output. Your output may differ:
Time 5 minutes ago: 20:50:45.239177
- Compare time_5minutes_later with time_5minutes_ago using the < operator:
>>> time_5minutes_later < time_5minutes_ago
We get the following output. Your output may differ:
False
- Compare time_5minutes_later with time_5minutes_ago using the > operator:
>>> time_5minutes_later > time_5minutes_ago
We get the following output. Your output may differ:
True
- Compare time_5minutes_later, time_now and time_5minutes_ago together using the > operator:
>> time_5minutes_later > time_now > time_5minutes_ago
We get the following output. Your output may differ:
True
How it works…
In step 1, you import date, datetime, and timedelta classes from the datetime module. In step 2, you fetch today's date using the today() classmethod provided by the class date and assign it to a new attribute, date_today. (A classmethod allows you to call a method directly on a class without creating an instance.) The return object is of type datetime.date. In step 3, you create a date, 5 days ahead of today, by adding a timedelta object, holding a duration of 5 days, to date_today. You assign this to a new attribute, date_5days_later. Similarly, in step 4, you create a date, 5 days ago and assign it to a new attribute date_5days_ago.
In step 5 and step 6, you compare date_5days_later and date_5days_ago using the > and < operators, respectively. The > operator returns True if the first operand holds a date ahead of that held by operand 2. Similarly, the < operator returns True if the second operand holds a date ahead of that held by operand 1. In step 7, you compare together all three date objects created so far. Note the outputs.
Step 8 to step 14 perform the same operations as step 2 to step 7, but this time on datetime.time objects—fetching current time, fetching a time 5 minutes ahead of the current time, fetching a time 5 minutes before the current time and comparing all the datetime.time objects which are created. The timedelta objects cannot be added to datetime.time objects directly to get time in the past or the future. To overcome this, you can add timedelta objects to datetime objects and then extract time from them using the time() method. You do this in step 10 and step 11.
There's more
The operations shown in this recipe on date and time objects can similarly be performed on datetime objects. Besides +, -, < and >, you can also use the following operators on datetime, date, and time objects:

This is not an exhaustive list of permissible operators. Refer to the official documentation on datetime module for more information: https://docs.python.org/3.8/library/datetime.html.