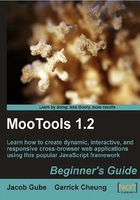
Time for action—create a chain of Fx.Tween methods
Let's see how to create a chain of Fx.Tween
methods by carrying out the following steps:
- Create a new HTML document like we always do. It should have the following code:
<html> <head> <script type="text/javascript" src="mootools-1.2.1-core-nc.js"> </script> <script type="text/javascript"> window.addEvent('domready', function() { }); </script> </head> <body> <div id="box" style="background-color: #00f; height:50px; width:50px;"> </div> </body> </html>
- Create a new object called
boxAnimation
, an instance of theFx.Tween
class, and giving it the target of our box element, which has anid
ofbox
, using the following code in ourdomready
event handler:var boxAnimation = new Fx.Tween( $('box') );
- Now that we have our
boxAnimation
object, let's apply the first method (moving it to the right by adjusting the left margin) by entering this line of code:boxAnimation.start( 'margin-left', 150 );
- We'll pause here and test our work. Save your document, and open it up in your web browser. You should see the following animation:
Then, you will see the following animation:
- Now, we'll add the second method, and we'll chain it to the previous method. That way, as soon as the first method gets completed, it will start our next method. Here's how to do it:
boxAnimation.start( 'margin-left', 150 ) .chain(function() { boxAnimation.start( 'width', 100 ); })
- You can save and test your work if you wish. If you execute our script, you'll see two consecutive animation effects move to the right and then increase width. This should be what you end up with:
- Let's add the rest of our chain methods, and this is what we should end up with:
.chain(function() { boxAnimation.start( 'width', 100 ); }) .chain(function() { boxAnimation.start('opacity', 0); }) .chain(function() { boxAnimation.start('opacity', 100); }) .chain(function() { boxAnimation.start('margin-left', 0); });
Here's the full sequence of the animation:
The next goes like this:
Then comes the following:
The next in the slide happens to be like the following:
The next slide looks like the following:
Lastly, the one aligned to the left:
What just happened?
Here, we just learned about how to create and instantiate an object of a MooTools class (Fx.Tween
), and learned how to use a method provided to us by another MooTools class (Chain
) using the .chain()
method.
By working through an example of how to chain methods in Fx.Tween
together (we chained the .start()
method which is actually in the Fx
class and is available to classes that extend Fx
, such as Fx.Morph)
, we're able to see the fundamental structure of MooTools. Its design is very class-based, and we work with objects by creating a blueprint, a template, and so on—oh here I go again!
We saw the concept of chaining in this example, and how we can trigger methods one after the other on the same object (in our example, boxAnimation)
.
It doesn't seem so special now, especially since we're just moving and resizing a box, but once we get into the thick of things, like making asynchronous server-side calls where we need to fire off functions in a sequence based on the state returned by our server, you'll see just exactly how great chaining is.
Have a go hero — doing more with chaining Fx.Tween methods
You can try to add or remove chained methods and experiment with different CSS properties. Perhaps you'll want to transition the background color to red (#F00) or adjust the height to double its size (to 100px).