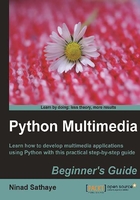
Time for action – connecting the widgets
You will notice several different widgets in the dialog. For example, the field which accepts the input image path or the output directory path is a QLineEdit
. The widget where image format is specified is a QCombobox
. On similar lines, the OK and Cancel buttons are QPushButton
. As an exercise, you can open up the thumbnailMaker.ui
file and click on each element to see the associated QT class from the Property Editor.
With this, let's learn how the widgets are connected.
- Open the file
ThumbnailMakerDialog.py
. The_connect
method of classThumbnailMakerDialog
is copied. The method is called in the constructor of this class.def _connect(self): """ Connect slots with signals. """ self.connect(self._dialog.inputFileDialogButton, SIGNAL("clicked()"), self._openFileDialog) self.connect(self._dialog.outputLocationDialogButton, SIGNAL("clicked()"), self._outputLocationPath) self.connect(self._dialog.okPushButton, SIGNAL("clicked()"), self._processImage) self.connect(self._dialog.closePushButton, SIGNAL("clicked()"), self.close) self.connect(self._dialog.aspectRatioCheckBox, SIGNAL('stateChanged(int)'), self._aspectRatioOptionChanged)
self._dialog
is an instance of classUi_ThumbnailMakerDialog
.self.connect
is the inherited method of Qt classQDialog
. Here, it takes the following arguments (QObject
,signal
,callable
), whereQObject
is any widget type (all inheritQObject
),signal
is the QTSIGNAL
that tells us about what event occurred andcallable
is any method handling this event.- For example, consider the highlighted lines of the code snippet. They connect the OK button to a method that handles image processing. The first argument ,
self._dialog.okPushButton
refers to the button widget defined in classUi_ThumbnailMakerDialog
. Referring toQPushButton
documentation, you will find there is a "clicked()
" signal that it can emit. The second argumentSIGNAL("clicked()")
tells Qt that we want to know when that button is clicked by the user. The third argument is the methodself._processImage
that gets called when this signal is emitted. - Similarly, you can review the other connections in this method. Each of these connects a widget to a method of the class
ThumbnailMakerDialog
.
What just happened?
We reviewed ThumbnailMakerDialog._connect()
method to understand how the UI elements are connected to various internal methods. The previous two sections helped us learn some preliminary concepts of GUI programming using QT.
Developing the image processing code
The previous sections were intended to get ourselves familiar with the application as an end user and to understand some basic aspects of the GUI elements in the application. With all necessary pieces together, let's focus our attention on the class that does all the main image processing in the application.
The class ThumbnailMaker
handles the pure image processing code. It defines various methods to achieve this. For example, the class methods such as _rotateImage
, _makeThumbnail
, and _resizeImage
manipulate the given image to accomplish rotation, thumbnail generation, and resizing respectively. This class accepts input from ThumbnailMakerDialog
. Thus, no QT related UI code is required here. If you want to use some other GUI framework to process input, you can do that easily. Just make sure to implement the public API methods defined in class ThumbnailMakerDialog
, as those are used by the ThumbnailMaker
class.