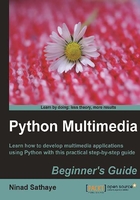
Time for action – cropping an image
This simple code snippet crops an image and applies some changes on the cropped portion.
- Download the file
Crop.png
from Packt website. The size of this image is400
x 400
pixels. You can also use your own image file. - Write the following code in a Python source file. Modify the path of the image file to an appropriate path.
import Image img = Image.open("C:\\images\\Crop.png") left = 0 upper = 0 right = 180 lower = 215 bbox = (left, upper, right, lower) img = img.crop(bbox) img.show()
- This will crop a region of the image bounded by
bbox
. The specification of the bounding box is identical to what we have seen in the Capturing screenshots section. The output of this example is shown in the following illustration.Original image (left) and its cropped region (right).
What just happened?
In the previous section, we used Image.crop
functionality to crop a region within an image and save the resultant image. In the next section, we will apply this while pasting a region of an image onto another.
Pasting
Pasting a copied or cut image onto another one is a commonly performed operation while processing images. Following is the simplest syntax to paste one image on another.
img = img.paste(image, box)
Here image
is an instance of class Image
and box
is a rectangular bounding box that defines the region of img
, where the image
will be pasted. The box
argument can be a 4-tupleError: Reference source not found
or a 2-tuple
. If a 4-tuple
box is specified, the size of the image to be pasted must be same as the size of the region. Otherwise, PIL will throw an error with a message ValueError:
images
do
not
match
. The 2-tuple
on the other hand, provides pixel coordinates of the upper-left corner of the region to be pasted.
Now look at the following line of code. It is a copy operation on an image.
img2 = img.copy(image)
The copy operation can be viewed as pasting the whole image onto a new image. This operation is useful when, for instance, you want to keep the original image unaltered and make alterations to the copy of the image.