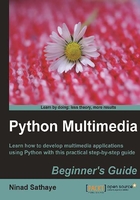
上QQ阅读APP看书,第一时间看更新
Time for action – creating a new image containing some text
As already stated, it is often useful to generate an image containing only some text or a common shape. Such an image can then be pasted onto another image at a desired angle and location. We will now create an image with text that reads, "Not really a fancy text!"
- Write the following code in a Python source file:
1 import Image 2 import ImageDraw 3 txt = "Not really a fancy text!" 4 size = (150, 50) 5 color = (0, 100, 0) 6 img = Image.new('RGB', size, color) 7 imgDrawer = ImageDraw.Draw(img) 8 imgDrawer.text((5, 20), txt)9 img.show()
- Let's analyze the code line by line. The first two lines import the necessary modules from PIL. The variable
txt
is the text we want to include in the image. On line 7, the new image is created usingImage.new
. Here we specify themode
andsize
arguments. The optionalcolor
argument is specified as atuple
with RGB values pertaining to the "dark green" color. - The
ImageDraw
module in PIL provides graphics support for anImage
object. The functionImageDraw.Draw
takes an image object as an argument to create aDraw
instance. In output code, it is calledimgDrawer
, as used on line 7. ThisDraw
instance enables drawing various things in the given image. - On line 8, we call the text method of the Draw instance and supply position (a
tuple
) and the text (stored in the stringtxt
) as arguments. - Finally, the image can be viewed using
img.show()
call. You can optionally save the image usingImage.save
method. The following screenshot shows the resultant image.
What just happened?
We just learned how to create an image from scratch. An empty image was created using the Image.new
method. Then, we used the ImageDraw
module in PIL to add text to this image.
Reading images from archive
If the image is part of an archived container, for example, a TAR archive, we can use the TarIO
module in PIL to open it and then call Image.open
to pass this TarIO
instance as an argument.