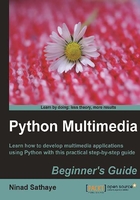
Built-in multimedia support
Python has a few built-in multimedia modules for application development. We will skim through some of these modules.
winsound
The winsound
module is available on the Windows platform. It provides an interface which can be used to implement fundamental audio-playing elements in the application. A sound can be played by calling PlaySound(sound,
flags)
. Here, the argument sound is used to specify the path of an audio file. If this parameter is specified as None
, the presently streaming audio (if any) is stopped. The second argument specifies whether the file to be played is a sound file or a system sound. The following code snippet shows how to play a wave formatted audio file using winsound
module.
from winsound import PlaySound, SND_FILENAME PlaySound("C:/AudioFiles/my_music.wav", SND_FILENAME )
This plays the sound file specified by the first argument to the function PlaySound
. The second argument, SND_FILENAME
, says that the first argument is an audio file. If the flag is set as SND_ALIAS
, it means the value for the first argument is a system sound from the registry.
audioop
This module is used for manipulating the raw audio data. One can perform several useful operations on sound fragments. For example, it can find the minimum and maximum values of all the samples within a sound fragment.
wave
The wave
module provides an interface to read and write audio files with WAV
file format. The following line of code opens a wav file.
import wave fil = wave.open('horn.wav', 'r')
The first argument of method open
is the location where the path to the wave file is specified. The second argument 'r' returns a Wave_read
object. This is the mode in which the audio file is opened, 'r
' or 'rb
' for read-only mode and 'w
' or 'wb
' for write-only mode.