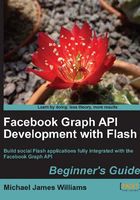
Time for action - displaying a Graph Object's connections
The Graph Object Renderer has the ability to show a list of all the object's connections, if that list is included as part of the Graph Object.
All we have to do is tell the Graph API to give us that list when we request a Graph Object; since our code for creating an instance of GraphObject
from a JSON copies all the properties of that JSON to the GraphObject
, this metadata will be included too. So, actually, all we need to do is add the metadata=1
flag to the end of the Graph URL that we request, and it'll do the rest for us.
We could do this by changing our request code as shown in the following excerpt:
public function CustomGraphContainerController (a_graphControlContainer:GraphControlContainer)
{
super(a_graphControlContainer);
var loader:URLLoader = new URLLoader();
var request:URLRequest = new URLRequest();
//Specify which Graph URL to load
request.url = "https://graph.facebook.com/PacktPub?metadata=1";
loader.addEventListener(Event.COMPLETE, onGraphDataLoadComplete);
//Start the actual loading process
loader.load(request);
}
There's a slightly more elegant way to do this, however, using a class called URLVariables
. In CustomGraphContainerController.as
, add a line to import this class:
import flash.net.URLVariables;
Now, modify the constructor as shown in the following lines of code:
public function CustomGraphContainerController (a_graphControlContainer:GraphControlContainer) { super(a_graphControlContainer); var loader:URLLoader = new URLLoader(); var request:URLRequest = new URLRequest(); var variables:URLVariables = new URLVariables(); //Specify which Graph URL to load request.url = "https://graph.facebook.com/PacktPub"; variables.metadata = 1; request.data = variables; loader.addEventListener(Event.COMPLETE, onGraphDataLoadComplete); //Start the actual loading process loader.load(request); }
As you can probably guess, setting variables.metadata = 1
is exactly the same as sticking ?metadata=1
on the end of the URL. Doing it this way takes a few more lines, but it makes it much easier to set different parameters, and keeps the parameters separate from the URL.
Anyway, compile the SWF and you should see the following screenshot:

Notice the new Connections bar at the bottom of the Renderer? Click on the Show button:

What just happened?
We can now see all of the connections of a Graph Object right there in its renderer. Of course, that's not very interesting unless we can see what's at the other end of each connection!
Ideally, whenever the user clicks a connection from the scrolling list, a new Graph List Renderer of that connection will be created and displayed.
To do this, we'd need to add a MouseEvent.CLICK
listener to the list, and use it to trigger a new URLLoader
request for the clicked connection.
Fortunately, all the UI code has already been provided elsewhere in the project; we just need to tap into that. To do this, we'll need to make use of what I call a Requestor.