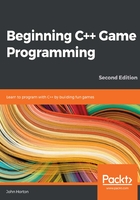
Handling death
Every game must end badly with either the player running out of time (which we have already handled) or getting squashed by a branch.
Detecting the player getting squashed is really simple. All we want to know is: does the last branch in the branchPositions array equal playerSide? If it does, the player is dead.
Add the following highlighted code that detects and executes when the player is squashed by a branch. We will talk about it later:
// Handle a flying log
if (logActive)
{
spriteLog.setPosition(
spriteLog.getPosition().x +
(logSpeedX * dt.asSeconds()),
spriteLog.getPosition().y +
(logSpeedY * dt.asSeconds()));
// Has the log reached the right-hand edge?
if (spriteLog.getPosition().x < -100 ||
spriteLog.getPosition().x > 2000)
{
// Set it up ready to be a whole new cloud next frame
logActive = false;
spriteLog.setPosition(800, 600);
}
}
// has the player been squished by a branch?
if (branchPositions[5] == playerSide)
{
// death
paused = true;
acceptInput = false;
// Draw the gravestone
spriteRIP.setPosition(525, 760);
// hide the player
spritePlayer.setPosition(2000, 660);
// Change the text of the message
messageText.setString("SQUISHED!!");
// Center it on the screen
FloatRect textRect = messageText.getLocalBounds();
messageText.setOrigin(textRect.left +
textRect.width / 2.0f,
textRect.top + textRect.height / 2.0f);
messageText.setPosition(1920 / 2.0f,
1080 / 2.0f);
}
} // End if(!paused)
/*
****************************************
Draw the scene
****************************************
*/
The first thing the preceding code does, after the player's demise, is set paused to true. Now, the loop will complete this frame and won't run the update part of the loop again until a new game is started by the player.
Then, we move the gravestone into position, near where the player was standing, and hide the player sprite off screen.
We set the String of messageText to "Squished!!" and then use the usual technique to center it on the screen.
You can now run the game and play it for real. The following screenshot shows the player's final score and their gravestone, as well as the SQUISHED message:

There is just one more problem to deal with. Is it just me, or is it a little bit quiet?