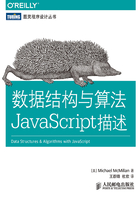
上QQ阅读APP看书,第一时间看更新
1.3 对象和面向对象编程
本书讨论到的数据结构都被实现为对象。JavaScript提供了多种方式来创建和使用对象。本节将要展示的这些技术,在本书用于创建对象,并用于创建和使用对象中的方法和属性。
对象通过如下方式创建:定义包含属性和方法声明的构造函数,并在构造函数后紧跟方法的定义。下面是一个检查银行账户对象的构造函数:
function Checking(amount) { this.balance = amount; //属性 this.deposit = deposit; //方法 this.withdraw = withdraw; //方法 this.toString = toString; //方法 }
this关键字用来将方法和属性绑定到一个对象的实例上。下面我们看看对于前面声明过的方法是如何定义的:
function deposit(amount) { this.balance += amount; } function withdraw(amount) { if (amount <= this.balance) { this.balance -= amount; } if (amount > this.balance) { print("Insufficient funds"); } } function toString() { return "Balance: " + this.balance; }
这里,我们又一次使用this关键字和balance属性,以便让JavaScript解释器知道我们引用的是哪个对象的balance属性。
例1-12 给出了Checking对象的完整定义和测试代码。
例1-12 定义和使用Checking对象
function Checking(amount) { this.balance = amount; this.deposit = deposit; this.withdraw = withdraw; this.toString = toString; } function deposit(amount) { this.balance += amount; } function withdraw(amount) { if (amount <= this.balance) { this.balance -= amount; } if (amount > this.balance) { print("Insufficient funds"); } } function toString() { return "Balance: " + this.balance; } var account = new Checking(500); account.deposit(1000); print(account.toString()); //Balance: 1500 account.withdraw(750); print(account.toString()); //余额:750 account.withdraw(800); //显示"余额不足" print(account.toString()); //余额:750