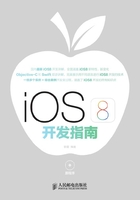
第7章 文本框和文本视图
在本章前面的内容中,已经创建了一个简单的应用程序,并学会了应用程序基础框架和图形界面基础框架。在本章的内容中,将详细介绍iOS应用中的基本构件,向读者讲解使用可编辑的文本框和文本视图的基本知识。
7.1 文本框(UITextField)
在iOS应用中,文本框和文本视图都是用于实现文本输入的,在本节的内容中,将首先详细讲解文本框的基本知识,为读者步入本书后面知识的学习打下基础
7.1.1 文本框基础
在iOS应用中,文本框(UITextField)是一种常见的信息输入机制,类似于Web表单中的表单字段。当在文本框中输入数据时,可以使用各种iOS键盘将其输入限制为数字或文本。和按钮一样,文本框也能响应事件,但是通常将其实现为被动(passive)界面元素,这意味着视图控制器可随时通过text属性读取其内容。
控件UITextField的常用属性如下所示。
(1)borderStyle属性:设置输入框的边框线样式。
(2)backgroundColor属性:设置输入框的背景颜色,使用其font属性设置字体。
(3)clearButtonMode属性:设置一个清空按钮,通过设置clearButtonMode可以指定是否以及何时显示清除按钮。此属性主要有如下几种类型。
UITextFieldViewModeAlways:不为空,获得焦点与没有获得焦点都显示清空按钮;
UITextFieldViewModeNever:不显示清空按钮;
UITextFieldViewModeWhileEditing:不为空,且在编辑状态时(及获得焦点)显示清空按钮;
UITextFieldViewModeUnlessEditing:不为空,且不在编译状态时(焦点不在输入框上)显示清空按钮。
(4)background属性:设置一个背景图片。
7.1.2 实战演练——在屏幕中显示一个文本输入框
在iOS应用中,可以使用控件UITextField在屏幕中显示一个文本输入框。UITextField通常用于外部数据输入,以实现人机交互。在本实例中,使用UItextField控件设置了一个文本输入框,并设置在框中显示提示文本“请输入信息”。

实例文件UIKitPrjPlaceholder.m的具体实现代码如下所示:
#import "UIKitPrjPlaceholder.h"
@implementation UIKitPrjPlaceholder
- (void)viewDidLoad {
[super viewDidLoad];
self.view.backgroundColor = [UIColor whiteColor];
UITextField* textField = [[[UITextField alloc] init]
autorelease];
textField.frame = CGRectMake( 20, 100, 280, 30 );
textField.borderStyle = UITextBorderStyleRoundedRect;
textField.contentVerticalAlignment =
UIControlContentVerticalAlignmentCenter;
textField.placeholder = @"请输入信息";
[self.view addSubview:textField];
}
@end
执行后的效果如图7-1所示。

图7-1 执行效果
7.1.3 实战演练——设置文本输入框的边框线样式
在本实例中,首先使用UITextField控件设置了4个文本输入框,然后使用borderStyle属性为这4个输入框设置了不同的边框线样式。

注意:为了便于对比,本书中的很多实例在同一个工程文件中实现。
实例文件 UIKitPrjSimple.m的具体实现代码如下所示:
#import "UIKitPrjSimple.h"
@implementation UIKitPrjSimple
- (void)dealloc {
[textFields_ release];
[super dealloc];
}
- (void)viewDidLoad {
[super viewDidLoad];
self.view.backgroundColor = [UIColor whiteColor];
UITextField* textField1 = [[[UITextField alloc] init] autorelease];
textField1.delegate = self;
textField1.frame = CGRectMake( 20, 20, 280, 30 );
textField1.borderStyle = UITextBorderStyleLine;
textField1.text = @"aaaaaaaaa";
textField1.returnKeyType = UIReturnKeyNext;
[self.view addSubview:textField1];
UITextField* textField2 = [[[UITextField alloc] init] autorelease];
textField2.delegate = self;
textField2.frame = CGRectMake( 20, 60, 280, 30 );
textField2.borderStyle = UITextBorderStyleBezel;
textField2.text = @"bbbbbbbbb";
textField2.returnKeyType = UIReturnKeyNext;
[self.view addSubview:textField2];
UITextField* textField3 = [[[UITextField alloc] init] autorelease];
textField3.delegate = self;
textField3.frame = CGRectMake( 20, 100, 280, 30 );
textField3.borderStyle = UITextBorderStyleRoundedRect;
textField3.text = @"ccccccccccc";
textField3.returnKeyType = UIReturnKeyNext;
[self.view addSubview:textField3];
UITextField* textField4 = [[[UITextField alloc] init] autorelease];
textField4.delegate = self;
textField4.frame = CGRectMake( 20, 140, 280, 30 );
textField4.borderStyle = UITextBorderStyleNone;
textField4.text = @"ddddddddddd";
textField4.returnKeyType = UIReturnKeyNext;
[self.view addSubview:textField4];
textFields_ = [[NSArray alloc] initWithObjects:textField1, textField2, textField3,
textField4, nil];
}
- (void)textFieldDidBeginEditing:(UITextField*)textField {
currentFieldIndex_ = [textFields_ indexOfObject:textField];
}
- (BOOL)textFieldShouldReturn:(UITextField*)textField {
if ( textFields_.count <= ++currentFieldIndex_ ) {
currentFieldIndex_ = 0;
}
UITextField* newField = [textFields_ objectAtIndex:currentFieldIndex_];
if ( [newField canBecomeFirstResponder] ) {
[newField becomeFirstResponder];
}
return YES;
}
@end
执行后的效果如图7-2所示。

图7-2 执行效果
7.1.4 实战演练——设置文本输入框的字体和颜色
在本实例中,首先使用UITextField控件设置了1个文本输入框,然后设置了输入框中默认显示的文本为“看我的字体和颜色”,并设置了文本的字体和整个输入框的背景颜色。

实例文件 UIKitPrjChangeColorAndFont.m的具体实现代码如下所示。
#import "UIKitPrjChangeColorAndFont.h"
@implementation UIKitPrjChangeColorAndFont
- (void)viewDidLoad {
[super viewDidLoad];
self.view.backgroundColor = [UIColor whiteColor];
UITextField* textField = [[[UITextField alloc] init] autorelease];
textField.frame = CGRectMake( 20, 100, 280, 50 );
textField.borderStyle = UITextBorderStyleBezel;
textField.backgroundColor = [UIColor blackColor];
//设置背景色
textField.textColor = [UIColor redColor];//设置文本颜色
textField.textAlignment = UITextAlignmentCenter;
textField.font = [UIFont systemFontOfSize:36];
//设置字体大小
textField.text = @"看我的字体和颜色";
[self.view addSubview:textField];
}
@end
执行后的效果如图7-3所示。

图7-3 执行效果
7.1.5 实战演练——在文本输入框中设置一个清空按钮
在本实例中,首先使用UITextField控件设置了4个文本输入框,然后分别为这4个输入框设置了不同类型的清空按钮。

实例文件 UIKitPrjClearButtonMode.m的具体实现代码如下所示:
#import "UIKitPrjClearButtonMode.h"
@implementation UIKitPrjClearButtonMode
- (void)dealloc {
[textFields_ release];
[super dealloc];
}
- (void)viewDidLoad {
[super viewDidLoad];
self.view.backgroundColor = [UIColor whiteColor];
UITextField* textField1 = [[[UITextField alloc] init] autorelease];
textField1.delegate = self;
textField1.clearsOnBeginEditing = YES;
textField1.frame = CGRectMake( 20, 20, 280, 30 );
textField1.borderStyle = UITextBorderStyleRoundedRect;
textField1.clearButtonMode = UITextFieldViewModeNever;
textField1.text = @"UITextFieldViewModeNever";
[self.view addSubview:textField1];
UITextField* textField2 = [[[UITextField alloc] init] autorelease];
textField2.delegate = self;
textField2.frame = CGRectMake( 20, 60, 280, 30 );
textField2.borderStyle = UITextBorderStyleRoundedRect;
textField2.clearButtonMode = UITextFieldViewModeWhileEditing;
textField2.text = @"UITextFieldViewModeWhileEditing";
[self.view addSubview:textField2];
UITextField* textField3 = [[[UITextField alloc] init] autorelease];
textField3.delegate = self;
textField3.frame = CGRectMake( 20, 100, 280, 30 );
textField3.borderStyle = UITextBorderStyleRoundedRect;
textField3.clearButtonMode = UITextFieldViewModeUnlessEditing;
textField3.text = @"UITextFieldViewModeUnlessEditing";
[self.view addSubview:textField3];
UITextField* textField4 = [[[UITextField alloc] init] autorelease];
textField4.delegate = self;
textField4.frame = CGRectMake( 20, 140, 280, 30 );
textField4.borderStyle = UITextBorderStyleRoundedRect;
textField4.clearButtonMode = UITextFieldViewModeAlways;
textField4.text = @"UITextFieldViewModeAlways";
[self.view addSubview:textField4];
textFields_ = [[NSArray alloc] initWithObjects:textField1, textField2, textField3,
textField4, nil];
}
- (BOOL)textFieldShouldClear:(UITextField*)textField {
NSLog( @"textFieldShouldClear:%@", textField.text );
return YES;
}
@end
执行后的效果如图7-4所示。

图7-4 执行效果
7.1.6 实战演练——为文本输入框设置背景图片
在本实例中,首先用 UITextField在屏幕中设置一个文本输入框,然后使用“textField.background =stretchableWhitePaper”语句为输入框设置了背景图片。

实例文件 UIKitPrjBackground.m 的具体实现代码如下所示:
#import "UIKitPrjBackground.h"
@implementation UIKitPrjBackground
- (void)viewDidLoad {
[super viewDidLoad];
self.view.backgroundColor = [UIColor whiteColor];
//导入背景图片,并设置成自动伸缩
UIImage* imageWhitePaper = [UIImage imageNamed:@"paper.png"];
UIImage* stretchableWhitePaper = [imageWhitePaper
stretchableImageWithLeftCapWidth:20 topCapHeight:20];
UIImage* imageGrayPaper = [UIImage imageNamed:@"paperGray.png"];
UIImage* stretchableGrayPaper = [imageGrayPaper stretchableImageWithLeftCapWidth:20
topCapHeight:20];
//创建UITextField实例
UITextField* textField = [[[UITextField alloc] init] autorelease];
textField.delegate = self;
textField.frame = CGRectMake( 20, 100, 280, 50 );
textField.background = stretchableWhitePaper;//设置背景图片
textField.disabledBackground = stretchableGrayPaper;
textField.text = @"有图片";
textField.textAlignment = UITextAlignmentCenter;
textField.contentVerticalAlignment = UIControlContentHorizontalAlignmentCenter;
[self.view addSubview:textField];
}
- (BOOL)textFieldShouldReturn:(UITextField*) textField {
textField.enabled = NO;
return YES;
}
@end
执行后的效果如图7-5所示。

图7-5 执行效果
7.1.7 实战演练——使用UITextField控件(基于Swift实现)
在本节的内容中,将通过一个具体实例的实现过程,详细讲解基于Swift语言使用UITextField控件的过程。

(1)打开Xcode 6,然后创建一个名为“UI007”的工程,工程的最终目录结构如图7-6所示。

图7-6 工程的目录结构
(2)编写文件RootViewController.swift,功能是在UITableView 控件中列表显示“self.items”中的条目,在“self.items”中只是包含了 UITextField控件。文件RootViewController.swift的具体实现代码如下所示:
import UIKit
class RootViewController : UIViewController, UITableViewDelegate, UITableViewDataSource
{
var tableView : UITableView?
var items : NSArray?
override func viewDidLoad()
{
self.title="Swift"
self.items = ["UITextField"]
self.tableView = UITableView(frame:self.view.frame,
style:UITableViewStyle.Plain)
self.tableView!.delegate = self
self.tableView!.dataSource = self
self.tableView!.registerClass(UITableViewCell.self, forCellReuseIdentifier:
"Cell")
self.view?.addSubview(self.tableView)
}
此时工程UI主界面的执行效果如图7-7所示。

图7-7 工程UI主界面的执行效果
(3)文件的 DetailViewController.swift功能是,当单击条目中的UITextField控件名称时,会在新界面中演示这个控件的功能。文件DetailViewController.swift的主要实现代码如下所示:
import UIKit
class DetailViewController:UIViewController,UIPickerViewDataSource,UIPickerViewDelegate
{
override func viewDidLoad()
{
self.view!.backgroundColor=UIColor.whiteColor()
if self.title == "UITextField"
{
var textField=UITextField(frame:CGRectMake(60.0, 120.0, 200.0, 30.0))
textField.backgroundColor = UIColor.lightGrayColor()
textField.placeholder = "input text"
self.view.addSubview(textField)
}
else
{}
}
到此为止,整个实例介绍完毕。单击UI主界面中“UITextField”列表项,在新界面中输入文本后的执行效果如图7-8所示。

图7-8 单击UI主界面中“UITextField”列表项后的界面
7.2 文本视图(UITextView)
文本视图(UITextView)与文本框类似,差别在于文本视图可显示一个可滚动和编辑的文本块,供用户阅读或修改。仅当需要的输入很多时,才应使用文本视图。
7.2.1 文本视图基础
在iOS应用中,UITextView是一个类。在Xcode中当使用IB给视图拖上去一个文本框后,选中文本框后可以在Attribute Inspector中设置其各种属性。
Attribute Inspector分为3部分,分别是Text Field、Control和View部分。我们重点看看Text Field 部分,Text Field部分有以下选项。
(1)Text:设置文本框的默认文本。
(2)Placeholder:可以在文本框中显示灰色的字,用于提示用户应该在这个文本框输入什么内容。当这个文本框中输入了数据时,用于提示的灰色的字将会自动消失。
(3)Background:设置背景。
(4)Disabled:若选中此项,用户将不能更改文本框内容。
(5)接下来是3个按钮,用来设置对齐方式。
(6)Border Style:选择边界风格。
(7)Clear Button:这是一个下拉菜单,你可以选择清除按钮什么时候出现,所谓清除按钮就是出一个现在文本框右边的小×,可以有以下选择:
Never appears:从不出现。
Appears while editing:编辑时出现。
Appears unless editing:编辑时不出现。
Is always visible:总是可见。
(8)Clear when editing begins:若选中此项,则当开始编辑这个文本框时,文本框中之前的内容会被清除掉。比如,先在这个文本框A中输入了“What”,之后去编辑文本框B,若再回来编辑文本框 A,则其中的“What”会被立即清除。
(9)Text Color:设置文本框中文本的颜色。
(10)Font:设置文本的字体与字号。
(11)Min Font Size:设置文本框可以显示的最小字体(不过我感觉没什么用)
(12)Adjust To Fit:指定当文本框尺寸减小时文本框中的文本是否也要缩小。选择它,可以使得全部文本都可见,即使文本很长。但是这个选项要跟Min Font Size配合使用,文本再缩小,也不会小于设定的 Min Font Size。
接下来的部分用于设置键盘如何显示。
(13)Captitalization: 设置大写。下拉菜单中有4个选项。
None:不设置大写。
Words:每个单词首字母大写,这里的单词指的是以空格分开的字符串。
Sentances:每个句子的第一个字母大写,这里的句子是以句号加空格分开的字符串。
All Characters:所有字母大写。
(14)Correction:检查拼写,默认是YES。
(15)Keyboard:选择键盘类型,比如全数字、字母和数字等。
(16)Return Key:选择返回键,可以选择Search、Return、Done等。
(17)Auto-enable Return Key:如选择此项,则只有至少在文本框输入一个字符后键盘的返回键才有效。
(18)Secure:当你的文本框用作密码输入框时,可以选择这个选项,此时,字符显示为星号。
在iOS应用中,可以使用UITextView在屏幕中显示文本,并且能够同时显示多行文本。UITextView的常用属性如下所示。
(1)textColor属性:设置文本的的颜色。
(2)font属性:设置文本的字体和大小。
(3)editable属性:如果设置为YES,可以将这段文本设置为可编辑的。
(4)textAlignment属性:设置文本的对齐方式,此属性有如下3个值:
UITextAlignmentRight:右对齐;
UITextAlignmentCenter:居中对齐;
UITextAlignmentLeft:左对齐。
7.2.2 实战演练——在屏幕中换行显示文本
在本实例中,使用控件UITextView在屏幕中同时显示了12行文本。并且设置了文本的颜色是白色,设置了字体大小是32。

实例文件 UIKitPrjTextView.m的具体代码如下所示。
#import "UIKitPrjTextView.h"
@implementation UIKitPrjTextView
- (void)viewDidLoad {
[super viewDidLoad];
UITextView* textView = [[[UITextView alloc] init] autorelease];
textView.frame = self.view.bounds;
textView.autoresizingMask =
UIViewAutoresizingFlexibleWidth | UIViewAutoresizingFlexibleHeight;
//textView.editable = NO; // 不可编辑
textView.backgroundColor = [UIColor blackColor]; // 背景为黑色
textView.textColor = [UIColor whiteColor]; // 字符为白色
textView.font = [UIFont systemFontOfSize:32]; // 字体的设置
textView.text = @"学习UITextView!\n"
"第2行\n"
"第3行\n"
"第4行\n"
"第5行\n"
"第6行\n"
"第7行\n"
"第8行\n"
"第9行\n"
"第10行\n"
"第11行\n"
"第12行\n";
[self.view addSubview:textView];
}
@end
执行后的效果如图7-9所示。

图7-9 执行效果
7.2.3 实战演练——在屏幕中显示可编辑的文本
在本实例中,使用控件UITextView在屏幕中显示了一段文本“亲们,可以编辑这一段文本。”,然后将其editable属性设置为YES。当单击“Edit”按钮后可以编辑这段文本,单击“Done”按钮后可以完成对这段文本的编辑操作。

实例文件 UIKitPrjEditableTextView.m的具体代码如下所示:
#import "UIKitPrjEditableTextView.h"
@implementation UIKitPrjEditableTextView
- (void)dealloc {
[textView_ release];
[super dealloc];
}
- (void)viewDidLoad {
[super viewDidLoad];
textView_ = [[UITextView alloc] init];
textView_.frame = self.view.bounds;
textView_.autoresizingMask = UIViewAutoresizingFlexibleWidth |
UIViewAutoresizingFlexibleHeight;
textView_.delegate = self;
textView_.text = @"亲们,可以编辑这一段文本。";
[self.view addSubview:textView_];
}
- (void)viewWillAppear:(BOOL)animated {
[super viewWillAppear:animated];
[self.navigationController setNavigationBarHidden:NO animated:YES];
[self.navigationController setToolbarHidden:NO animated:YES];
}
- (void)viewDidAppear:(BOOL)animated {
[super viewDidAppear:animated];
[self textViewDidEndEditing:textView_]; // 画面显示时设置为非编辑模式
}
- (void)viewWillDisappear:(BOOL)animated {
[super viewWillDisappear:animated];
[textView_ resignFirstResponder]; // 画面跳转时设置为非编辑模式
}
- (void)textViewDidBeginEditing:(UITextView*)textView {
static const CGFloat kKeyboardHeight = 216.0;
// 按钮设置为[完成]
self.navigationItem.rightBarButtonItem =
[[[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemDone
target:self action:@selector(doneDidPush)] autorelease];
[UIView beginAnimations:nil context:nil];
[UIView setAnimationDuration:0.3];
// 缩小UITextView以免被键盘挡住
CGRect textViewFrame = textView.frame;
textViewFrame.size.height = self.view.bounds.size.height - kKeyboardHeight;
textView.frame = textViewFrame;
// 工具条位置上移
CGRect toolbarFrame = self.navigationController.toolbar.frame;
toolbarFrame.origin.y =
self.view.window.bounds.size.height - toolbarFrame.size.height - kKeyboardHeight;
self.navigationController.toolbar.frame = toolbarFrame;
[UIView commitAnimations];
}
- (void)textViewDidEndEditing:(UITextView*)textView {
// 按钮设置为[编辑]
self.navigationItem.rightBarButtonItem =
[[[UIBarButtonItem alloc] initWithBarButtonSystemItem:UIBarButtonSystemItemEdit
target:self action:@selector(editDidPush)] autorelease];
[UIView beginAnimations:nil context:nil];
[UIView setAnimationDuration:0.3];
// 恢复UITextView的尺寸
textView.frame = self.view.bounds;
// 恢复工具条的位置
CGRect toolbarFrame = self.navigationController.toolbar.frame;
toolbarFrame.origin.y =
self.view.window.bounds.size.height - toolbarFrame.size.height;
self.navigationController.toolbar.frame = toolbarFrame;
[UIView commitAnimations];
}
- (void)editDidPush {
[textView_ becomeFirstResponder];
}
- (void)doneDidPush {
[textView_ resignFirstResponder];
}
@end
执行后的效果如图7-10所示。单击“Edit”按钮后可以编辑这段文字,如图7-11所示。

图7-10 执行效果

图7-11 编辑界面
7.2.4 实战演练——设置屏幕中文本的对齐方式
在本实例中,使用控件UITextView在屏幕中显示了一段文本“此文本可编辑”,然后在工具条中添加了4个按钮。其中通过按钮“alignment”可以控制文本的对齐方式,通过按钮“Selection”可以获得文本的范围。

实例文件 UIKitPrjWorkingWithTheSelection.m的具体实现流程如下所示:
#import "UIKitPrjWorkingWithTheSelection.h"
static const CGFloat kKeyboardHeight = 216.0;
@implementation UIKitPrjWorkingWithTheSelection
- (void)dealloc {
[textView_ release];
[super dealloc];
}
- (void)viewDidLoad {
[super viewDidLoad];
// UITextView的追加
textView_ = [[UITextView alloc] init];
textView_.frame = self.view.bounds;
textView_.autoresizingMask = UIViewAutoresizingFlexibleWidth |
UIViewAutoresizingFlexibleHeight;
textView_.text = @"此文本可编辑。";
[self.view addSubview:textView_];
// 在工具条中追加按钮
UIBarButtonItem* hasTextButton =
[[[UIBarButtonItem alloc] initWithTitle:@"hasText"
style:UIBarButtonItemStyleBordered
target:self
action:@selector(hasTextDidPush)] autorelease];
UIBarButtonItem* selectionButton =
[[[UIBarButtonItem alloc] initWithTitle:@"selection"
style:UIBarButtonItemStyleBordered
target:self
action:@selector(selectionDidPush)] autorelease];
UIBarButtonItem* alignmentButton =
[[[UIBarButtonItem alloc] initWithTitle:@"alignment"
style:UIBarButtonItemStyleBordered
target:self
action:@selector(alignmentDidPush)] autorelease];
UIBarButtonItem* scrollButton =
[[[UIBarButtonItem alloc] initWithTitle:@"top"
style:UIBarButtonItemStyleBordered
target:self
action:@selector(scrollDidPush)] autorelease];
NSArray* buttons = [NSArray arrayWithObjects:hasTextButton, selectionButton,
alignmentButton, scrollButton, nil];
[self setToolbarItems:buttons animated:YES];
}
- (void)viewDidAppear:(BOOL)animated {
[super viewDidAppear:animated];
// 调整工具条位置
[UIView beginAnimations:nil context:nil];
[UIView setAnimationDuration:0.3];
textView_.frame =
CGRectMake( 0, 0, self.view.bounds.size.width, self.view.bounds.size.height -
kKeyboardHeight );
CGRect toolbarFrame = self.navigationController.toolbar.frame;
toolbarFrame.origin.y =
self.view.window.bounds.size.height - toolbarFrame.size.height - kKeyboardHeight;
self.navigationController.toolbar.frame = toolbarFrame;
[UIView commitAnimations];
[textView_ becomeFirstResponder]; //< 画面显示时显示键盘
}
- (void)viewWillDisappear:(BOOL)animated {
[super viewWillDisappear:animated];
// 恢复工具条
[UIView beginAnimations:nil context:nil];
[UIView setAnimationDuration:0.3];
textView_.frame = self.view.bounds;
CGRect toolbarFrame = self.navigationController.toolbar.frame;
toolbarFrame.origin.y = self.view.window.bounds.size.height -
toolbarFrame.size.height;
self.navigationController.toolbar.frame = toolbarFrame;
[UIView commitAnimations];
[textView_ resignFirstResponder]; // 画面隐藏时隐藏键盘
}
- (void)hasTextDidPush {
UIAlertView* alert = [[[UIAlertView alloc] init] autorelease];
if ( textView_.hasText ) {
alert.message = @"textView_.hasText = YES";
} else {
alert.message = @"textView_.hasText = NO";
}
[alert addButtonWithTitle:@"OK"];
[alert show];
}
- (void)selectionDidPush {
UIAlertView* alert = [[[UIAlertView alloc] init] autorelease];
alert.message = [NSString stringWithFormat:@"location = %d, length = %d",
textView_.selectedRange.location, textView_.selectedRange.length];
[alert addButtonWithTitle:@"OK"];
[alert show];
}
- (void)alignmentDidPush {
textView_.editable = NO;
if ( UITextAlignmentRight <
++textView_.textAlignment ) {
textView_.textAlignment = UITextAlignmentLeft;
}
textView_.editable = YES;
}
- (void)scrollDidPush {
// NSRange scrollRange = NSMakeRange( 0, 1 );
[textView_ scrollRangeToVisible:NSMakeRange( 0,
1 )];
}
@end
执行后的效果如图7-12所示。

图7-12 执行效果
7.2.5 实战演练——使用UITextView控件(基于Swift实现)
在本节的内容中,将通过一个具体实例的实现过程,详细讲解基于Swift语言使用UITextView控件的过程。

(1)打开Xcode 6,然后创建一个名为“UI014”的工程,工程的最终目录结构如图7-13所示。

图7-13 工程的目录结构
(2)编写文件RootViewController.swift,功能是在UITableView 控件中列表显示“self.items”中的条目,在“self.items”中只是包含了UITextView控件。文件RootViewController.swift的具体实现代码如下所示:
import UIKit
class RootViewController : UIViewController, UITableViewDelegate,
UITableViewDataSource
{
var tableView : UITableView?
var items: NSArray?
override func viewDidLoad()
{
self.title="Swift"
self.items = ["UITextView"]
self.tableView=UITableView(frame:self.view.frame,style:UITableViewStyle.Plain)
self.tableView!.delegate = self
self.tableView!.dataSource = self
self.tableView!.registerClass(UITableViewCell.self, forCellReuseIdentifier:
"Cell")
self.view?.addSubview(self.tableView)
}
此时工程UI主界面的执行效果如图7-14所示。

图7-14 工程UI主界面的执行效果
(3)文件的 DetailViewController.swift功能是,当单击条目中的UITextView控件名称时,会在新界面中演示这个控件的功能。文件DetailViewController.swift的主要实现代码如下所示:
import UIKit
class DetailViewController:UIViewController,UIPickerViewDataSource,UIPickerViewDelegate
{
override func viewDidLoad()
{
self.view!.backgroundColor=UIColor.whiteColor()
if self.title == "UITextView"
{
var textView=UITextView(frame:CGRectMake(10.0, 120.0, 300.0, 200.0))
textView.backgroundColor =
UIColor.lightGrayColor()
textView.editable = false
textView.font =
UIFont.systemFontOfSize(20)
textView.text = "Swift is an innovative
new programming language for Cocoa and Cocoa Touch.
Writing code is interactive and fun, the syntax is
concise yet expressive, and apps run lightning-fast.
Swift is ready for your next iOS and OS X project
— or for addition into your current app — because
Swift code works side-by-side with Objective-C."
self.view.addSubview(textView)
}
else
{}
}
到此为止,整个实例介绍完毕。单击UI主界面中“UITextView”列表项后的执行效果如图7-15所示。

图7-15 单击UI主界面中“UITextView”列表项后的界面